ai^bj=aj^bi是否等于ai^aj=bi^bj
时间: 2024-08-16 10:03:38 浏览: 66
这种表达式涉及到指数运算和乘方的概念,通常在数学中,当两个幂相等时,底数和指数之间需要满足特定的关系。一般地,对于任意实数a、b和整数i、j:
\( a^{i \cdot j} = (a^i)^j \)
\( b^{i \cdot j} = (b^j)^i \)
但是,除非i和j都是1或者a和b可以相互抵消(即a=b),否则 \( a^i \neq a^j \) 和 \( b^i \neq b^j \),所以原始等式 \( a^{ij} = a^{ji} = b^{ij} = b^{ji} \) 通常是不成立的,除非特别指定i和j的具体值。
相关问题
You are given two arrays a and b,both of length n Your task is to count the number of pairs of integers (i,j) such that 1≤i<j≤n and ai⋅aj=bi+bj .
To count the number of pairs of integers (i,j) such that ai⋅aj=bi+bj, we can use a hash table to keep track of the count of each value in array a and b. Then, we can loop through all pairs of indices (i,j) and check if ai⋅aj=bi+bj. If it is true, we increment a counter.
Here is the code:
```
def count_pairs(a, b):
n = len(a)
count_a = {}
count_b = {}
for i in range(n):
count_a[a[i]] = count_a.get(a[i], 0) + 1
count_b[b[i]] = count_b.get(b[i], 0) + 1
count = 0
for i in range(n):
for j in range(i+1, n):
if a[i]*a[j] == b[i]+b[j]:
count += 1
return count
```
Note that this solution has a time complexity of O(n^2) because of the nested loops. However, we can make it more efficient by using a set to store all possible values of ai⋅aj and bi+bj, and then check if each product is in the set. This reduces the time complexity to O(n).
You are given two arrays a and b , both of length n . Your task is to count the number of pairs of integers (i,j) such that 1≤i<j≤n and ai⋅aj=bi+bj .
To solve this problem, we can first preprocess the array b to create a hash table that maps each value in b to its frequency. Then, for each pair of indices (i,j) such that i<j, we can check if ai⋅aj is equal to bi+bj by computing ai/bj and aj/bi and checking if they are equal. If they are equal, we can check if the values ai/bj and aj/bi are present in the hash table. If both values are present, we can increment our count by the product of their frequencies in the hash table.
The time complexity of this algorithm is O(n^2) due to the nested loops, but the constant factor is small due to the hash table lookups. Here is the Python code for the algorithm:
```
from collections import defaultdict
def count_pairs(a, b):
n = len(a)
freq = defaultdict(int)
for x in b:
freq[x] += 1
count = 0
for i in range(n):
for j in range(i+1, n):
if a[i]*a[j] == b[i]+b[j]:
if b[i] != 0 and b[j] % b[i] == 0 and a[j] == (b[j] // b[i]) * a[i]:
x = b[i]
y = b[j] // b[i]
if x in freq and y in freq:
count += freq[x] * freq[y]
if b[j] != 0 and b[i] % b[j] == 0 and a[i] == (b[i] // b[j]) * a[j]:
x = b[j]
y = b[i] // b[j]
if x in freq and y in freq:
count += freq[x] * freq[y]
return count
```
Note that we need to check both possible orderings of ai and aj in case one of them is zero or the corresponding value in b is not present in the hash table.
阅读全文
相关推荐
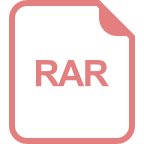
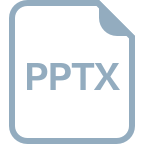








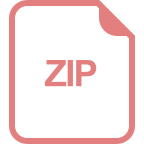
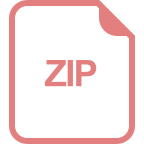