ai^bj=aj^bi
时间: 2024-08-16 11:03:37 浏览: 95
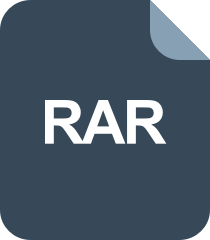
diaodu.rar_Algorithm A5 GSM_Java 8_diaodu_动态规划 java
这个表达式看起来像是涉及到了指数运算。"ai^bj" 和 "aj^bi" 表示两个数 \(a_i\) 和 \(a_j\) 的指数形式,其中 i 和 j 分别是它们的指数。如果这里的 "^" 表示乘方运算,那么等式的含义就是第一个数的第二个幂等于第二个数的第一个幂。数学上,这种相等通常只在两个底数相同时成立,即 \(a_i = a_j\) 时,\(a_i^{b_j} = a_j^{b_i}\)。
然而,如果没有特定的上下文,比如这是指数函数的性质或者是某个特殊规则的一部分,我们无法给出确切的解释。如果这是一个数学谜题或者代数问题的一部分,通常会有一个具体的条件来说明如何处理这种情况。
阅读全文
相关推荐
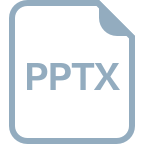









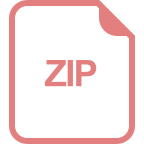
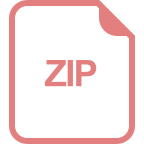