optics聚类算法python代码
时间: 2023-11-12 16:59:55 浏览: 110
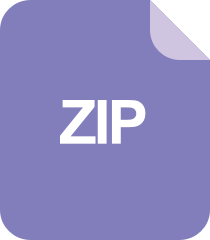
聚类分析OPTICS算法python实现
好的,以下是一个简单的Python实现的optics聚类算法代码:
```python
import numpy as np
from sklearn.neighbors import NearestNeighbors
class OPTICS:
def __init__(self, eps, min_samples):
self.eps = eps
self.min_samples = min_samples
def fit(self, X):
self.X = X
self.n_samples = X.shape[0]
self.visited_ = np.zeros(self.n_samples, dtype=bool)
self.ordering_ = []
self.core_distances_ = np.zeros(self.n_samples)
self.reachability_distances_ = np.ones(self.n_samples) * np.inf
self.process()
def process(self):
self.tree_ = NearestNeighbors(radius=self.eps, algorithm='ball_tree').fit(self.X)
for point_idx in range(self.n_samples):
if not self.visited_[point_idx]:
self.visited_[point_idx] = True
self.ordering_.append(point_idx)
neighbors_idx = self.tree_.radius_neighbors([self.X[point_idx]], return_distance=False)[0]
n_neighbors = len(neighbors_idx)
if n_neighbors >= self.min_samples:
self.core_distances_[point_idx] = np.max(self.tree_.kneighbors([self.X[point_idx]], n_neighbors=self.min_samples)[0])
self.update(neighbors_idx, point_idx)
self.ordering_ = np.array(self.ordering_)
self.cluster_ordering_ = np.argsort(self.reachability_distances_)
self.reachability_distances_[self.reachability_distances_ == np.inf] = -1
def update(self, neighbors_idx, point_idx):
core_dist = self.core_distances_[point_idx]
for neighbor in neighbors_idx:
if not self.visited_[neighbor]:
new_reach_dist = max(core_dist, np.linalg.norm(self.X[point_idx] - self.X[neighbor]))
if np.isinf(self.reachability_distances_[neighbor]):
self.reachability_distances_[neighbor] = new_reach_dist
else:
self.reachability_distances_[neighbor] = min(new_reach_dist, self.reachability_distances_[neighbor])
def extract_clusters(self):
clusters = []
idx = 0
while idx < self.n_samples:
if self.reachability_distances_[self.cluster_ordering_[idx]] == -1:
idx += 1
continue
cluster = [self.cluster_ordering_[idx]]
while True:
idx += 1
if idx == self.n_samples:
break
if self.reachability_distances_[self.cluster_ordering_[idx]] == -1:
continue
if self.reachability_distances_[self.cluster_ordering_[idx]] > self.eps:
break
cluster.append(self.cluster_ordering_[idx])
clusters.append(cluster)
return clusters
```
阅读全文
相关推荐
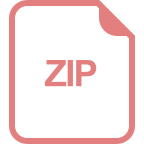
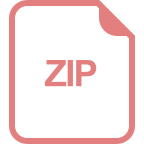















