写一个在 C 语言中实现将文件CodeFile.txt以紧凑格式显示在终端上(每行 50 个代码),并且同时将其写入文件CodePrint.txt中的示例代码
时间: 2024-12-12 12:23:56 浏览: 5
在 C 语言中,你可以使用标准输入输出流以及`fgets()`函数逐行读取文件,并结合循环控制每一行显示和写入。下面是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
#define LINE_LENGTH 50
int main() {
FILE *input_file = fopen("CodeFile.txt", "r");
if (input_file == NULL) {
perror("Error opening input file");
return 1;
}
FILE *output_file = fopen("CodePrint.txt", "w");
if (output_file == NULL) {
perror("Error creating output file");
fclose(input_file);
return 1;
}
char line[LINE_LENGTH + 1]; // Buffer for each line
size_t len;
while ((len = fgets(line, sizeof(line), input_file)) != 0) { // Read until EOF
if (len > LINE_LENGTH) { // If line is longer than 50 characters
line[LINE_LENGTH] = '\n'; // Truncate and add newline
} else {
line[len] = '\0'; // Null terminate the line if it's within length
}
printf("%s", line); // Display on terminal
fprintf(output_file, "%s\n", line); // Write to output file
}
fclose(input_file);
fclose(output_file);
printf("\nFinished processing.\n");
return 0;
}
```
这个程序首先打开两个文件,一个是读取源文件"CodeFile.txt",另一个是写入处理后的结果到"CodePrint.txt"。然后通过循环读取源文件的一行并检查其长度,如果超过50字符则截断并在末尾添加换行符。最后,它会打印到控制台并在输出文件中写入每行。
阅读全文
相关推荐
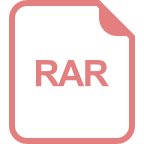
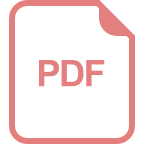
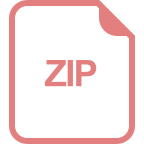















