C语言如何随机生成稀疏矩阵代码
时间: 2024-11-17 22:00:56 浏览: 65
在C语言中,生成稀疏矩阵通常不会像常规二维数组那样直接存储每个元素,因为稀疏矩阵大部分元素为0,这样会造成内存浪费。一种常见的方法是使用邻接列表(Adjacency List)或者三元组表示法(Triplets)。以下是使用邻接列表创建稀疏矩阵的一种简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int row; // 行索引
int col; // 列索引
double value; // 值
} SparseMatrixEntry;
// 稀疏矩阵结构体
typedef struct {
int **entries; // 邻接列表,行索引指向列索引的数组
int numRows;
int numCols;
int capacity;
} SparseMatrix;
// 创建新的稀疏矩阵
SparseMatrix *createSparseMatrix(int numRows, int numCols) {
SparseMatrix *matrix = (SparseMatrix *) malloc(sizeof(SparseMatrix));
matrix->numRows = numRows;
matrix->numCols = numCols;
matrix->capacity = numRows * numCols;
matrix->entries = (int **) malloc(matrix->capacity * sizeof(int *));
for (int i = 0; i < matrix->capacity; i++) {
matrix->entries[i] = NULL;
}
return matrix;
}
// 添加矩阵元素
void addEntry(SparseMatrix *matrix, int row, int col, double value) {
if (row >= matrix->numRows || col >= matrix->numCols) {
printf("Invalid index\n");
return;
}
// 找到对应位置的节点并添加值
int *list = matrix->entries[row];
while (list != NULL && list[0] != col) {
list = list->next;
}
if (list == NULL) {
list = (int *) malloc(sizeof(int) + sizeof(double));
list[0] = col;
list->next = matrix->entries[row];
matrix->entries[row] = list;
} else {
list->next->value += value;
}
}
// 示例用法
int main() {
SparseMatrix *sparseMat = createSparseMatrix(5, 6);
addEntry(sparseMat, 0, 1, 4.0); // 矩阵 [0,1] 的值为 4.0
// 添加更多条目...
// 销毁时记得释放资源
free(sparseMat->entries);
free(sparseMat);
return 0;
}
阅读全文
相关推荐
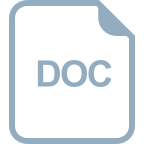
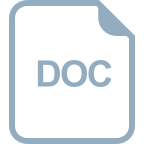
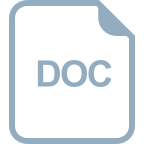



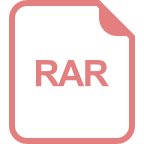



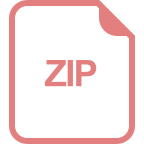
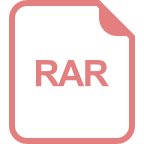
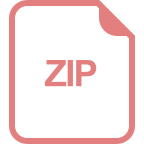
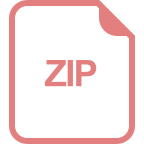
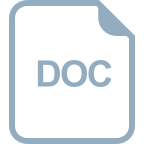
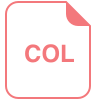


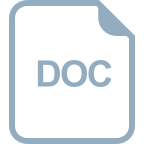