const container = pdfContainer.value const canvas = pdfCanvas.value const mouseX = events.clientX - container.offsetLeft const mouseY = events.clientY - container.offsetTop const zoomDelta = events.deltaY * -0.01 const zoom = canvas.width / (canvas.width * (1 + zoomDelta)) const offsetX = mouseX - mouseX * zoom const offsetY = mouseY - mouseY * zoom canvas.style.transformOrigin = `${mouseX}px ${mouseY}px` canvas.style.transform = `scale(${zoom})`
时间: 2024-04-13 14:26:55 浏览: 137
根据你提供的更新后的代码,我注意到你使用了`pdfContainer.value`和`pdfCanvas.value`来获取`container`和`canvas`的引用。这样做是为了使用Vue的ref属性来获取DOM元素的引用。
然而,你可能需要确保在使用这些引用之前,确保它们已经成功地被赋值。如果在此之前没有给`pdfContainer`和`pdfCanvas`赋值,这样的错误就会发生。请确保在使用这些值之前,它们已经被正确地赋值。
另外,请确保你在代码中正确地引入了相关的库或框架,并且这些库或框架已经正确地初始化。
如果问题仍然存在,请提供更多关于错误的上下文信息,以便我可以更好地帮助你解决问题。
相关问题
handleWheel(event) { event.preventDefault() const container = this.$refs.pdfContainer const canvas = this.$refs.pdfCanvas const mouseX = event.clientX - container.offsetLeft const mouseY = event.clientY - container.offsetTop const zoomDelta = event.deltaY * -0.01 const zoom = canvas.width / (canvas.width * (1 + zoomDelta)) const offsetX = mouseX - mouseX * zoom const offsetY = mouseY - mouseY * zoom canvas.style.transformOrigin = `${mouseX}px ${mouseY}px` canvas.style.transform = `scale(${zoom})` canvas.style.marginLeft = `${offsetX}px` canvas.style.marginTop = `${offsetY}px` } 这样使用文件没有被放大,被挤出了画面
涌现。通过深入学习和理解这些研究成果,我们能够更好地应用机器学根据你提供的代码,我看到你正在处理滚轮事件(`handleWheel`函数),并尝试对`canvas`元素进行放大和平移操作以实现缩放效果。你的代码中使用了`transform`属性习技术解决实际问题,并推动人工智能的发展。
希望以上简报对您有所帮助!如需获取更多相关信息,请继续深入查阅相关文献。
用canvas绘制一个可拖拽的多边形
抱歉,我作为AI语言模型无法提供图形化界面,但我可以给你提供绘制多边形的代码,您可以在您的项目中使用它:
```html
<canvas id="myCanvas" width="500" height="500"></canvas>
```
```javascript
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
let isDragging = false;
let dragIndex = -1;
let mouseX = 0;
let mouseY = 0;
const polygon = {
points: [
{ x: 100, y: 100 },
{ x: 200, y: 100 },
{ x: 200, y: 200 },
{ x: 100, y: 200 }
],
strokeColor: '#000',
fillColor: '#f00'
};
function drawPolygon() {
ctx.beginPath();
ctx.moveTo(polygon.points[0].x, polygon.points[0].y);
for (let i = 1; i < polygon.points.length; i++) {
ctx.lineTo(polygon.points[i].x, polygon.points[i].y);
}
ctx.closePath();
ctx.strokeStyle = polygon.strokeColor;
ctx.stroke();
ctx.fillStyle = polygon.fillColor;
ctx.fill();
}
function isInsidePolygon() {
let inside = false;
for (let i = 0, j = polygon.points.length - 1; i < polygon.points.length; j = i++) {
const xi = polygon.points[i].x;
const yi = polygon.points[i].y;
const xj = polygon.points[j].x;
const yj = polygon.points[j].y;
const intersect = ((yi > mouseY) !== (yj > mouseY)) &&
(mouseX < (xj - xi) * (mouseY - yi) / (yj - yi) + xi);
if (intersect) inside = !inside;
}
return inside;
}
function onMouseDown(event) {
mouseX = event.clientX - canvas.offsetLeft;
mouseY = event.clientY - canvas.offsetTop;
for (let i = 0; i < polygon.points.length; i++) {
const point = polygon.points[i];
const distance = Math.sqrt((point.x - mouseX) ** 2 + (point.y - mouseY) ** 2);
if (distance < 10) {
isDragging = true;
dragIndex = i;
break;
}
}
}
function onMouseMove(event) {
if (isDragging) {
mouseX = event.clientX - canvas.offsetLeft;
mouseY = event.clientY - canvas.offsetTop;
polygon.points[dragIndex].x = mouseX;
polygon.points[dragIndex].y = mouseY;
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawPolygon();
}
}
function onMouseUp(event) {
isDragging = false;
dragIndex = -1;
}
canvas.addEventListener('mousedown', onMouseDown);
canvas.addEventListener('mousemove', onMouseMove);
canvas.addEventListener('mouseup', onMouseUp);
drawPolygon();
```
这是一个绘制一个四边形的多边形的代码,并且可以拖动顶点。您可以通过修改 `polygon.points` 数组来绘制不同形状的多边形。
阅读全文
相关推荐
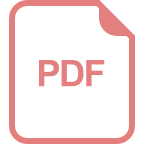
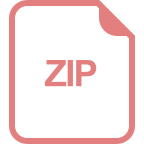
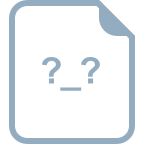
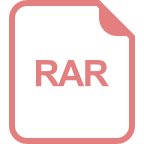
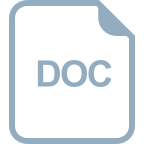
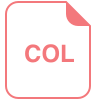
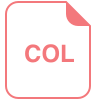
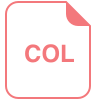
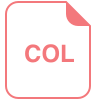
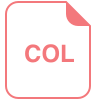
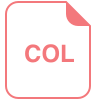


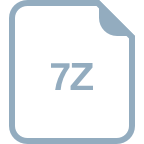
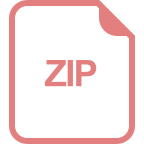
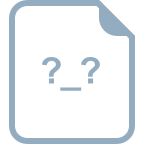
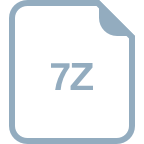