dijkstra matlab
时间: 2023-11-02 10:00:33 浏览: 48
Dijkstra's algorithm is a popular algorithm for finding the shortest path between two nodes in a graph. In MATLAB, you can implement Dijkstra's algorithm using a combination of data structures and loops. Here's a simple example of Dijkstra's algorithm implemented in MATLAB:
```matlab
function [distances, paths] = dijkstra(graph, startNode)
% Initialize the distances and paths
numNodes = size(graph, 1);
distances = inf(1, numNodes);
paths = cell(1, numNodes);
% Set the distance of the start node to 0
distances(startNode) = 0;
% Create a priority queue to keep track of the unvisited nodes
unvisited = 1:numNodes;
while ~isempty(unvisited)
% Find the node with the minimum distance
[~, index] = min(distances(unvisited));
currentNode = unvisited(index);
% Remove the current node from the unvisited set
unvisited(index) = [];
% Update the distances and paths of the neighboring nodes
neighbors = find(graph(currentNode, :) > 0);
for i = 1:length(neighbors)
neighbor = neighbors(i);
distance = distances(currentNode) + graph(currentNode, neighbor);
if distance < distances(neighbor)
distances(neighbor) = distance;
paths{neighbor} = [paths{currentNode}, neighbor];
end
end
end
end
```
To use this implementation, you need to provide a weighted adjacency matrix `graph` representing the connectivity and distances between nodes. The `startNode` parameter specifies the node from which you want to find the shortest paths.
After calling the `dijkstra` function, you will get two outputs: `distances` and `paths`. The `distances` vector contains the shortest distances from the start node to all other nodes in the graph, and the `paths` cell array contains the shortest paths themselves.
Note that this is just a basic example, and there are many ways to optimize and improve the efficiency of Dijkstra's algorithm.
相关推荐
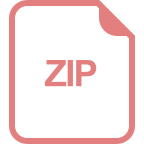














