NET8 实现自定义认证过滤器,在控制器上使用自定义特性ID,登录成功后获取角色,根据角色获取特性ID,如果用户角色中包含该ID则认证成功,都则认证失败。 使用Session
时间: 2024-10-02 22:04:04 浏览: 23
在.NET 8(实际上应该是.NET Core 3.x版本)中,要实现这种自定义认证过滤器,你需要编写一个自定义特性(Attribute)和一个控制器上的过滤器。这里是一个基本的步骤:
1. **创建自定义特性**:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using Microsoft.AspNetCore.Mvc.Filters;
using Microsoft.AspNetCore.Session;
using Microsoft.AspNetCore.Http;
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Method)]
public class CustomAuthorizeAttribute : AuthorizeAttribute
{
public string[] RequiredRoles { get; set; }
public CustomAuthorizeAttribute(params string[] roles)
{
RequiredRoles = roles;
}
protected override void HandleUnauthorizedRequest(AuthorizationFilterContext context)
{
var session = context.HttpContext.Session;
var userIdentity = context.HttpContext.User.Identity;
// 检查会话中是否存在登录信息和角色集合
var loginInfo = session.GetString("LoginInfo") as string[];
if (loginInfo != null && loginInfo.Contains(userIdentity.Name) &&
loginInfo.Last() == userIdentity.GetRoles().FirstOrDefault())
{
// 如果会话中的角色包含所需的角色,则认为认证通过
if (RequiredRoles.All(role => loginInfo.Contains(role)))
base.HandleUnauthorizedRequest(context);
else
{
// 认证失败,可以根据需要返回错误页面或重定向
context.Result = Unauthorized();
}
}
else
{
// 登录信息不在会话中或角色不匹配,直接返回Unauthorized
context.Result = Unauthorized();
}
}
}
```
2. **在控制器上使用自定义特性**:
将`CustomAuthorizeAttribute`应用到需要验证的角色的控制器或方法上:
```csharp
[CustomAuthorize(Roles = "Admin")]
public class AdminController : Controller
{
// 控制器方法...
}
```
3. **初始化会话**:
在应用程序启动时或请求处理之前,可以设置会话存储,如使用内存存储:
```csharp
app.UseSessions(new SessionOptions
{
// 设置会话选项,如过期时间等
// 存储位置可以在Startup.cs配置文件中指定
SaveMode = SaveMode.ServerOnly,
ExpireTimeSpan = TimeSpan.FromMinutes(30),
});
// 可能还需要开启中间件
app.UseSession();
```
阅读全文
相关推荐
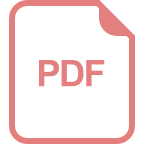
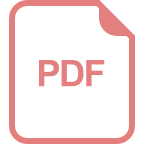
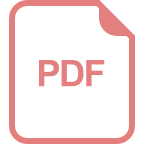















