def get_windict(words,window):
时间: 2024-11-09 12:17:58 浏览: 5
`get_windict` 函数看起来像是一个用于文本处理或自然语言处理中的函数,它接受两个参数:`words` 和 `window`。这个函数很可能用于生成词典或者构建词语的上下文窗口。
`words` 可能是一个单词列表或者是句子的分词结果,而 `window` 则通常是一个整数,表示每个单词应该考虑的前后词汇范围,即所谓的“滑动窗口”大小。例如,如果 window 设置为2,那么对于列表中的每个单词,函数会创建一个包含该单词及其前后两个单词(如果有)的键值对作为字典的一部分。
具体实现可能会像这样:
```python
def get_windict(words, window):
word_dict = {}
for i in range(len(words) - window + 1): # 避免索引越界
context = words[i:i+window] # 获取当前窗口内的单词序列
if context not in word_dict: # 如果这个词组不在字典里,添加进去
word_dict[context] = None # 或者设置初始值
return word_dict
相关问题
import os import jieba import gensim from langconv import Converter from gensim.corpora import WikiCorpus # 定义LabeledLineSentence类 class LabeledLineSentence(object): def __init__(self, wkc): self.wkc = wkc self.wkc.metadata = True def __iter__(self): for content, (page_id, title) in self.wkc.get_texts(): yield gensim.models.doc2vec.TaggedDocument( words=[w for c in content for w in jieba.cut( Converter('zh-hans').convert(c))], tags=[title]) # 代码7-5 def train(): zh_name = 'data/zh-latest-pages-articles.xml.bz2' wkc = WikiCorpus(zh_name, lemmatize=False, dictionary={}) documents = LabeledLineSentence(wkc) model = gensim.models.Doc2Vec(documents, dm=0, dbow_words=1, size=192, window=8, min_count=19, iter=5, workers=8) # 使用Doc2Vec训练段落向量 model.save('tmp/zhwk_news.doc2vec') if __name__ == '__main__': if os.path.exists('tmp/zhwk_news.doc2vec') == False: print('开始训练模型') train() print('模型训练完毕') # 代码7-6 model = gensim.models.Doc2Vec.load('tmp/zhwk_news.doc2vec') print(model.dv.similarity('番茄', '西红柿')) # 相似度为0.55 print(model.dv.similarity('货车', '卡车')) # 相似度为0.78
这段代码使用了gensim库中的Doc2Vec模型来训练中文文本,训练时使用了中文维基百科的语料库,使用了jieba分词和langconv进行繁简体转换。训练完成后,保存在本地,并使用gensim中的Doc2Vec.load方法进行加载。最后,通过model.dv.similarity方法计算两个词的相似度,其中'番茄'和'西红柿'的相似度是0.55,'货车'和'卡车'的相似度是0.78。
請你將這個製作成GUI窗口import random # 生命次数 lives = 3 # 神秘单词, 随机选择 words = ['pizza', 'fairy', 'teeth', 'shirt', 'otter', 'plane'] secret_word = random.choice(words) # print(secret_word) clue = list('?????') heart_symbol = u'\u2764' guessed_word_correctly = False def update_clue(guessed_letter, secret_word, clue): index = 0 while index < len(secret_word): if guessed_letter == secret_word[index]: clue[index] = guessed_letter index = index + 1 while lives > 0: print(clue) print('剩余生命次数: ' + heart_symbol * lives) guess = input('猜测字母或者是整个单词: ') if guess == secret_word: guessed_word_correctly = True break if guess in secret_word: update_clue(guess, secret_word, clue) else: print('错误。你丢了一条命\n') lives = lives - 1 if guessed_word_correctly: print('你赢了! 秘密单词是 ' + secret_word) else: print('你输了! 秘密单词是 ' + secret_word)
好的,我可以帮你将这段代码转换成GUI窗口程序。你需要安装Python的GUI库,比如Tkinter或者PyQt等。
下面是代码的GUI版本:
```python
import random
import tkinter as tk
# 初始化界面
window = tk.Tk()
window.title("猜单词游戏")
window.geometry("400x300")
# 生命次数
lives = 3
# 神秘单词
words = ['pizza', 'fairy', 'teeth', 'shirt', 'otter', 'plane']
secret_word = random.choice(words)
# 提示信息
clue = list('?????')
heart_symbol = u'\u2764'
# 是否猜对单词
guessed_word_correctly = False
# 更新提示信息
def update_clue(guessed_letter, secret_word, clue):
index = 0
while index < len(secret_word):
if guessed_letter == secret_word[index]:
clue[index] = guessed_letter
index = index + 1
# 处理玩家猜测
def handle_guess(guess_entry, lives_label, clue_label):
global lives, guessed_word_correctly
guess = guess_entry.get().lower()
if guess == secret_word:
guessed_word_correctly = True
result_label.config(text="你赢了! 秘密单词是 " + secret_word)
return
if guess in secret_word:
update_clue(guess, secret_word, clue)
else:
lives = lives - 1
lives_label.config(text="剩余生命次数: " + heart_symbol * lives)
if lives == 0:
result_label.config(text="你输了! 秘密单词是 " + secret_word)
return
clue_label.config(text=" ".join(clue))
guess_entry.delete(0, tk.END)
# 创建界面组件
guess_label = tk.Label(window, text="猜测字母或者是整个单词:")
guess_label.pack()
guess_entry = tk.Entry(window)
guess_entry.pack()
guess_button = tk.Button(window, text="猜测", command=lambda: handle_guess(guess_entry, lives_label, clue_label))
guess_button.pack()
clue_label = tk.Label(window, text=" ".join(clue))
clue_label.pack()
lives_label = tk.Label(window, text="剩余生命次数: " + heart_symbol * lives)
lives_label.pack()
result_label = tk.Label(window, text="")
result_label.pack()
# 开始界面循环
window.mainloop()
```
运行上述代码,将会弹出一个GUI窗口,你可以在窗口中猜测单词,程序会自动更新提示信息和剩余生命次数。当你猜对单词或者生命次数用完时,程序会显示相应的结果。
阅读全文
相关推荐
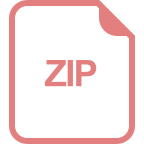
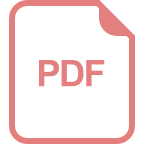
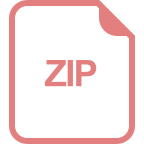
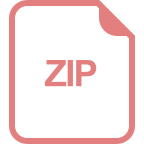
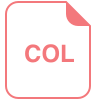
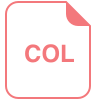
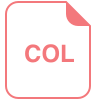
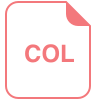
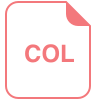
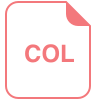
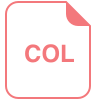
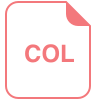
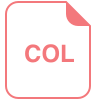
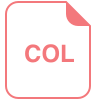
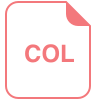
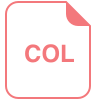

