蚁群算法求解旅行商问题代码
时间: 2025-01-04 12:23:18 浏览: 13
### 蚁群算法实现旅行商问题(TSP)代码示例
#### Python版本的蚁群算法解决TSP问题
```python
import numpy as np
import matplotlib.pyplot as plt
class AntColonyOptimization:
def __init__(self, distances, n_ants=10, n_best=20, n_iterations=1000, decay=0.95, alpha=1, beta=1):
self.distances = distances
self.pheromone = np.ones(self.distances.shape) / len(distances)
self.all_inds = range(len(distances))
self.n_ants = n_ants
self.n_best = n_best
self.n_iterations = n_iterations
self.decay = decay
self.alpha = alpha
self.beta = beta
def run(self):
shortest_path = None
all_time_shortest_path = ("placeholder", np.inf)
for i in range(self.n_iterations):
all_paths = self.gen_all_paths()
self.spread_pheronome(all_paths, self.n_best)
self.pheromone *= self.decay
current_shortest_path = min(all_paths, key=lambda x: x[1])
if current_shortest_path[1] < all_time_shortest_path[1]:
all_time_shortest_path = current_shortest_path
shortest_path = current_shortest_path
return all_time_shortest_path
def spread_pheronome(self, all_paths, n_best):
sorted_paths = sorted(all_paths, key=lambda x: x[1])
for path, dist in sorted_paths[:n_best]:
for move in zip(path[:-1], path[1:]):
self.pheromone[move] += 1.0 / self.distances[move]
def gen_path_dist(self, path):
total_dist = 0
for ele in zip(path[:-1], path[1:]):
total_dist += self.distances[ele]
return total_dist
def gen_all_paths(self):
all_paths = []
for i in range(self.n_ants):
path = self.gen_path(0)
all_paths.append((path, self.gen_path_dist(path)))
return all_paths
def gen_path(self, start):
path = []
visited = set()
visited.add(start)
prev = start
for _ in range(len(self.distances) - 1):
move = self.pick_move(self.pheromone[prev], self.distances[prev], visited)
path.append(move)
prev = move
visited.add(move)
path.append(start)
return path
def pick_move(self, pheromone, dist, visited):
pheromone = np.copy(pheromone)
pheromone[list(visited)] = 0
row = pheromone ** self.alpha * ((1.0 / dist) ** self.beta)
norm_row = row / row.sum()
move = np_choice(self.all_inds, 1, p=norm_row)[0]
return move
def main():
city_coordinates = [
(60, 200), (84, 253), (70, 25), (100, 100),
(200, 160), (150, 5), (50, 50), (150, 300),
(300, 200), (200, 100), (300, 100), (150, 75)
]
distance_matrix = calculate_distance(city_coordinates)
aco = AntColonyOptimization(distance_matrix, n_ants=20, n_best=20,
n_iterations=1000, decay=0.95, alpha=1, beta=1)
shortest_path = aco.run()
print(f'Shortest Path found by ACO is {shortest_path}')
plot_tsp(shortest_path)
def calculate_distance(coordinates):
number_of_cities = len(coordinates)
matrix = [[0 for col in range(number_of_cities)]
for row in range(number_of_cities)]
for idx_from, coord_from in enumerate(coordinates):
for idx_to, coord_to in enumerate(coordinates):
dx, dy = coord_from[0]-coord_to[0], coord_from[1]-coord_to[1]
dist = round(np.sqrt(dx*dx + dy*dy)) # Euclidean Distance
matrix[idx_from][idx_to] = dist
return np.array(matrix)
def plot_tsp(solution):
cities = solution[0]
coords = [(60, 200), (84, 253), (70, 25), (100, 100),
(200, 160), (150, 5), (50, 50), (150, 300),
(300, 200), (200, 100), (300, 100), (150, 75)]
plt.figure(figsize=(10, 7))
xs, ys = [], []
for c in cities:
xs.append(coords[c][0])
ys.append(coords[c][1])
xs.append(xs[0]) # Return to the starting point.
ys.append(ys[0])
plt.plot(xs, ys, 'o-r')
plt.show()
if __name__ == "__main__":
main()
```
此段代码展示了如何利用蚁群算法来找到给定一组城市的最短路径[^1]。通过定义`AntColonyOptimization`类,实现了蚂蚁个体的行为逻辑及其群体协作机制,并最终找到了一条较优的城市访问顺序。
阅读全文
相关推荐



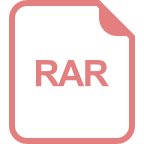












