建立一个包括头结点和3个结点(4,2,1)的单链表,实现单链表建立的基本操作:插入,删除,查找操作
时间: 2024-10-12 17:15:56 浏览: 30
要创建一个包含头节点和三个元素(4, 2, 1)的单链表,并实现基本的操作如插入、删除和查找,我们首先需要定义链表结构(Node),然后创建一个单链表类,提供相应的接口。
**链表结构(Node):**
```c
// Node structure for the singly linked list
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域,指向下一个节点
} Node;
```
**单链表类(LinkedList):**
```c
// LinkedList class with insertion, deletion, and search operations
typedef struct LinkedList {
Node* head; // 头节点指针
} LinkedList;
// 创建链表并初始化头节点
LinkedList* createLinkedList(int arr[], int size) {
LinkedList* list = (LinkedList*)malloc(sizeof(LinkedList));
list->head = NULL;
if (size > 0) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[0];
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Node* temp = list->head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
// 插入剩余元素
for (int i = 1; i < size; i++) {
newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = NULL;
temp = list->head;
while (temp->next != NULL && temp->next->data < arr[i]) {
temp = temp->next;
}
newNode->next = temp->next;
temp->next = newNode;
}
}
return list;
}
// 在链表末尾插入节点
void insertAtEnd(LinkedList* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
Node* temp = list->head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 删除指定值的节点(如果存在)
Node* deleteNode(LinkedList* list, int value) {
if (list->head == NULL) {
return NULL;
}
Node* temp = list->head;
Node* prev = NULL;
if (temp != NULL && temp->data == value) {
list->head = temp->next;
free(temp);
return temp;
}
while (temp != NULL && temp->data != value) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
return NULL;
}
prev->next = temp->next;
free(temp);
return prev;
}
// 查找节点是否存在
bool searchNode(LinkedList* list, int value) {
Node* current = list->head;
while (current != NULL) {
if (current->data == value) {
return true;
}
current = current->next;
}
return false;
}
```
现在你可以用这些函数来创建链表、插入、删除和查找元素了。例如:
```c
int main() {
int arr[] = {4, 2, 1};
int size = sizeof(arr) / sizeof(arr[0]);
LinkedList* list = createLinkedList(arr, size);
// 插入操作
insertAtEnd(list, 5);
// 删除操作
Node* deletedNode = deleteNode(list, 2);
// 查找操作
bool found = searchNode(list, 1);
// 清理内存
Node* node = list->head;
while (node != NULL) {
Node* nextTemp = node->next;
free(node);
node = nextTemp;
}
free(list);
return 0;
}
```
阅读全文
相关推荐
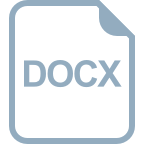
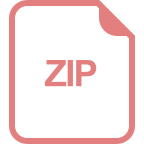
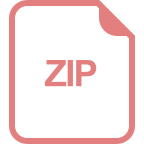















