输出所有出栈的可能C#
时间: 2024-10-20 13:19:18 浏览: 14
在C#中,如果你想要模拟一个栈的数据结构,并输出所有出栈的可能顺序,你可以创建一个栈并在循环中不断弹出元素并打印出来。这里是一个简单的例子,假设我们有一个整数栈:
```csharp
using System;
using System.Collections.Generic;
public class StackExample
{
private List<int> stack = new List<int>();
// 入栈操作
public void Push(int value)
{
stack.Add(value);
}
// 出栈操作
public int Pop()
{
if (stack.Count == 0) throw new InvalidOperationException("Stack is empty");
return stack.RemoveAt(stack.Count - 1);
}
// 打印出栈的所有可能顺序
public void PrintAllPossibleOutpus()
{
for (int i = stack.Count - 1; i >= 0; i--)
{
Console.WriteLine($"Output #{i + 1}: {stack[i]}");
}
}
}
public class Program
{
public static void Main(string[] args)
{
var stackObj = new StackExample();
// 假设这里先入栈了几个数字
stackObj.Push(1);
stackObj.Push(2);
stackObj.Push(3);
stackObj.PrintAllPossibleOutpus();
}
}
```
在这个程序中,`Push`方法用于向栈中添加元素,`Pop`方法用于移除并返回栈顶元素。`PrintAllPossibleOutpus`方法会遍历栈,从最后一个元素开始逐个出栈并打印。
阅读全文
相关推荐
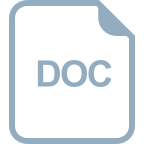
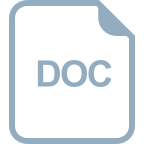
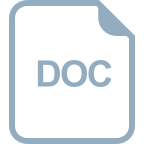
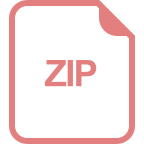
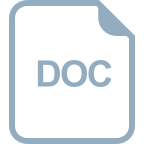
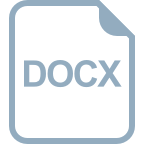
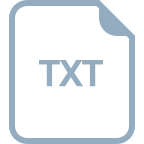
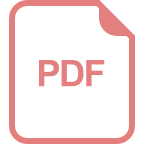
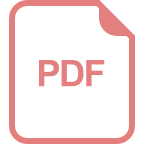
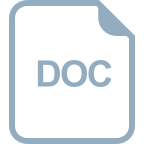
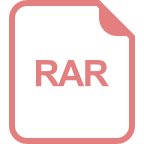
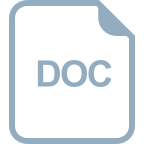
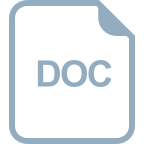





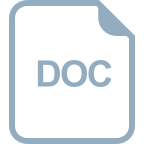