c#链栈实现数制的转换
时间: 2023-10-28 15:06:40 浏览: 41
以下是使用 C# 链栈实现数制转换的示例代码:
```csharp
using System;
// 定义链栈节点
class Node
{
public int data; // 节点数据
public Node next; // 下一个节点指针
public Node(int data)
{
this.data = data;
this.next = null;
}
}
// 定义链栈类
class LinkStack
{
private Node top; // 栈顶节点指针
public LinkStack()
{
this.top = null;
}
// 判断栈是否为空
public bool IsEmpty()
{
return this.top == null;
}
// 入栈
public void Push(int data)
{
Node newNode = new Node(data);
newNode.next = this.top;
this.top = newNode;
}
// 出栈
public int Pop()
{
if (this.IsEmpty())
{
Console.WriteLine("栈已空!");
return -1;
}
int data = this.top.data;
this.top = this.top.next;
return data;
}
// 获取栈顶元素
public int GetTop()
{
if (this.IsEmpty())
{
Console.WriteLine("栈已空!");
return -1;
}
return this.top.data;
}
}
class Program
{
static void Main(string[] args)
{
int num, baseNum;
Console.Write("请输入一个十进制整数:");
num = int.Parse(Console.ReadLine());
Console.Write("请输入要转换的进制数(2-9):");
baseNum = int.Parse(Console.ReadLine());
LinkStack stack = new LinkStack();
while (num > 0)
{
int remainder = num % baseNum;
stack.Push(remainder);
num /= baseNum;
}
Console.Write("转换后的结果为:");
while (!stack.IsEmpty())
{
Console.Write(stack.Pop());
}
Console.WriteLine();
}
}
```
运行程序,输入一个十进制整数和要转换的进制数,程序会使用链栈进行进制转换并输出结果。
相关推荐
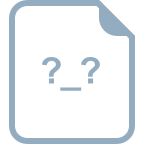
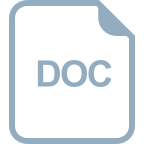














