一个4行4列的二维整数数组matrix,生成一个新的4行4列的二维数组,数组的元素为matrix对应位置元素在matrix中出现的次数,c++
时间: 2024-10-09 14:06:58 浏览: 27
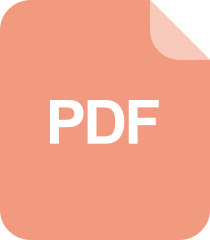
C语言数组:C语言数组定义、二维数组、动态数组、字符串数组_C语言中文网1
在C++中,你可以遍历给定的4x4二维整数数组`matrix`,统计每个元素出现的次数,并创建一个新的数组来存储这些计数。以下是一个简单的示例:
```cpp
#include <vector>
#include <unordered_map>
std::vector<std::vector<int>> countOccurrences(const std::vector<std::vector<int>>& matrix) {
std::vector<std::vector<int>> counts(4, std::vector<int>(4, 0)); // 新的4x4数组用于存储计数
// 使用一个哈希表来跟踪每个元素及其出现次数
std::unordered_map<int, int> elementCounts;
// 遍历原矩阵
for (size_t i = 0; i < 4; ++i) {
for (size_t j = 0; j < 4; ++j) {
elementCounts[matrix[i][j]]++; // 对每个元素计数
}
}
// 更新新矩阵的元素值为原元素的计数
for (size_t i = 0; i < 4; ++i) {
for (size_t j = 0; j < 4; ++j) {
counts[i][j] = elementCounts.find(matrix[i][j])->second; // 获取并设置计数值
}
}
return counts;
}
// 测试函数
int main() {
std::vector<std::vector<int>> matrix = {{1, 2, 2, 3},
{2, 2, 3, 3},
{2, 3, 3, 3},
{3, 3, 3, 3}};
std::vector<std::vector<int>> countedMatrix = countOccurrences(matrix);
// 打印新矩阵
for (const auto& row : countedMatrix) {
for (int count : row) {
std::cout << count << " ";
}
std::cout << "\n";
}
return 0;
}
```
这段代码会统计`matrix`中每个整数出现的次数,并将结果存储在一个新的4x4矩阵`countedMatrix`中。注意这里使用了`std::unordered_map`作为哈希表来提高查找和更新计数的效率。
阅读全文
相关推荐
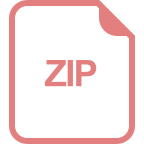
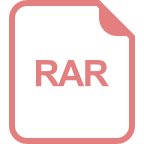












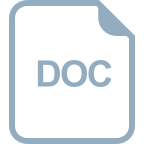
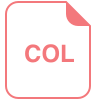


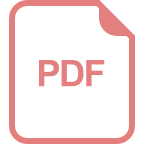