设计复数类myComplex,复数的实部和虚部均为double类型,并且放在private段;重载复数类的构造函数,及复制构造函数;重载复数运算+,-,*,/;重载复数流运算符<<,>>。同时实现返回复数实部、虚部、模的成员函数。
时间: 2024-12-22 16:28:04 浏览: 3
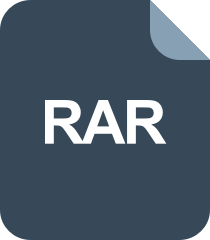
复数类加减法运算符重载成员函数

设计一个名为`myComplex`的复数类,如下所示:
```cpp
class myComplex {
private:
double real; // 实部
double imag; // 虚部
public:
// 构造函数
myComplex(double r = 0, double i = 0) : real(r), imag(i) {} // 默认构造
myComplex(const myComplex& other) : real(other.real), imag(other.imag) {} // 复制构造函数
// 操作符重载
myComplex operator+(const myComplex& b) const { // 加法
return myComplex(real + b.real, imag + b.imag);
}
myComplex operator-(const myComplex& b) const { // 减法
return myComplex(real - b.real, imag - b.imag);
}
myComplex operator*(const myComplex& b) const { // 乘法
return myComplex(real * b.real - imag * b.imag, real * b.imag + imag * b.real);
}
myComplex operator/(const myComplex& b) const { // 除法
double denominator = b.real * b.real + b.imag * b.imag;
if (denominator != 0)
return myComplex((real * b.real + imag * b.imag) / denominator,
(imag * b.real - real * b.imag) / denominator);
else
throw std::invalid_argument("Division by zero is not allowed.");
}
// 流运算符重载
friend std::ostream& operator<<(std::ostream& os, const myComplex& c) { // 输出
os << c.real << " + " << c.imag << "i";
return os;
}
friend std::istream& operator>>(std::istream& is, myComplex& c) { // 输入
char unit;
is >> c.real >> unit >> c.imag;
if (unit == 'i')
c.imag *= -1; // 如果输入的是 'i' 后面跟着数字,则表示虚部为负
return is;
}
// 成员函数
double getReal() const { return real; } // 获取实部
double getImaginary() const { return imag; } // 获取虚部
double magnitude() const { // 返回复数的模
return sqrt(real * real + imag * imag);
}
};
```
阅读全文
相关推荐
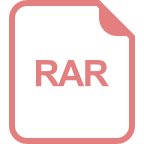
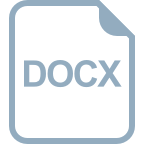


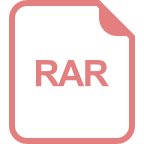
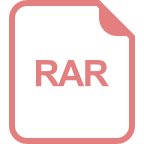












