c语言timer定时器
时间: 2023-12-02 20:41:14 浏览: 78
C语言中可以使用定时器来实现一些定时任务,例如定时执行某个函数或者在一定时间后执行某个操作。下面是两种实现定时器的方法:
1. 使用结构体和函数指针实现定时器
可以使用结构体来定义一个定时器,其中包含了定时器的超时时间、重复次数和超时后需要执行的函数指针。然后使用一个定时器管理器来管理所有的定时器,定时器管理器会不断地检查每个定时器是否超时,如果超时则执行相应的函数。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/time.h>
typedef struct timer {
struct timeval timeout; // 超时时间
int repeat; // 重复次数
void (*callback)(void); // 超时后需要执行的函数指针
} Timer;
void timer_init(Timer *timer, int sec, int usec, int repeat, void (*callback)(void)) {
gettimeofday(&timer->timeout, NULL);
timer->timeout.tv_sec += sec;
timer->timeout.tv_usec += usec;
timer->repeat = repeat;
timer->callback = callback;
}
int timer_expired(Timer *timer) {
struct timeval now; gettimeofday(&now, NULL);
return timer->timeout.tv_sec < now.tv_sec || (timer->timeout.tv_sec == now.tv_sec && timer->timeout.tv_usec <= now.tv_usec);
}
void timer_run(Timer *timer) {
timer->callback();
if (timer->repeat > 0) {
timer->repeat--;
gettimeofday(&timer->timeout, NULL);
timer->timeout.tv_sec += 1;
}
}
int main() {
Timer timer1, timer2;
timer_init(&timer1, 1, 0, 3, &timer1_callback);
timer_init(&timer2, 2, 0, 0, &timer2_callback);
while (1) {
if (timer_expired(&timer1)) {
timer_run(&timer1);
}
if (timer_expired(&timer2)) {
timer_run(&timer2);
break;
}
}
return 0;
}
void timer1_callback() {
printf("timer1 callback\n");
}
void timer2_callback() {
printf("timer2 callback\n");
}
```
2. 使用time.h库函数实现定时器
C语言中的time.h库提供了一些函数来获取当前时间和计算时间差等功能,可以使用这些函数来实现定时器。下面是一个简单的例子:
```c
#include <stdio.h>
#include <time.h>
int main() {
time_t start_time, current_time;
time(&start_time);
printf("start time: %ld\n", start_time);
while (1) {
time(¤t_time);
if (current_time - start_time >= 10) {
printf("current time: %ld, 10 seconds passed, exit\n", current_time);
break;
}
}
return 0;
}
```
相关推荐
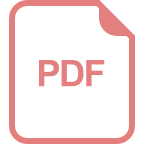
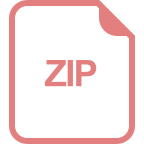














