请用C语言编写 已知二叉树的先序序列,输出层序遍历序列。 输入格式: 输入在一行中给出二叉树的先序序列。*表示空格 输出格式: 输出共一行,是二叉树的层序遍历序列。
时间: 2024-06-13 07:04:35 浏览: 80
已知二叉树的先序序列,输出层序遍历序列的C语言代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct TreeNode *BinTree;
struct TreeNode {
char Data;
BinTree Left;
BinTree Right;
};
typedef struct QueueRecord *Queue;
struct QueueRecord {
int Capacity;
int Front;
int Rear;
int Size;
BinTree *Array;
};
Queue CreateQueue(int MaxSize) {
Queue Q = (Queue)malloc(sizeof(struct QueueRecord));
Q->Array = (BinTree*)malloc(sizeof(BinTree) * MaxSize);
Q->Capacity = MaxSize;
Q->Front = 0;
Q->Rear = -1;
Q->Size = 0;
return Q;
}
int IsFull(Queue Q) {
return Q->Size == Q->Capacity;
}
int IsEmpty(Queue Q) {
return Q->Size == 0;
}
void Enqueue(Queue Q, BinTree X) {
if (IsFull(Q)) {
printf("队列已满\n");
return;
}
Q->Rear = (Q->Rear + 1) % Q->Capacity;
Q->Array[Q->Rear] = X;
Q->Size++;
}
BinTree Dequeue(Queue Q) {
if (IsEmpty(Q)) {
printf("队列为空\n");
return NULL;
}
BinTree X = Q->Array[Q->Front];
Q->Front = (Q->Front + 1) % Q->Capacity;
Q->Size--;
return X;
}
BinTree CreateBinTree() {
char ch;
scanf("%c", &ch);
if (ch == '*') {
return NULL;
}
BinTree T = (BinTree)malloc(sizeof(struct TreeNode));
T->Data = ch;
T->Left = CreateBinTree();
T->Right = CreateBinTree();
return T;
}
void LevelOrderTraversal(BinTree BT) {
if (!BT) {
return;
}
Queue Q = CreateQueue(MAXSIZE);
Enqueue(Q, BT);
while (!IsEmpty(Q)) {
BinTree T = Dequeue(Q);
printf("%c ", T->Data);
if (T->Left) {
Enqueue(Q, T->Left);
}
if (T->Right) {
Enqueue(Q, T->Right);
}
}
}
int main() {
BinTree BT = CreateBinTree();
LevelOrderTraversal(BT);
return 0;
}
```
阅读全文
相关推荐
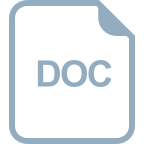
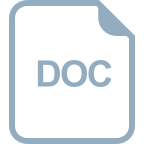
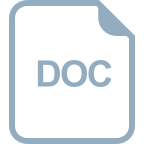















