设计BF算法,完成如下功能: 1)建立目标串s=“abcabcabdabba”和模式串t=“abcabd”。 2)采用BF算法求t在s中的位置。用c语言写
时间: 2024-10-19 11:11:27 浏览: 42
Bloom Filter(布隆过滤器)是一种空间效率极高的概率型数据结构,用于判断某个元素是否在一个集合中。然而,它并不适合查找精确的位置信息,如您提到的BF算法(Boyer-Moore字符串搜索算法),这是一种高效的字符串匹配算法。
Boyer-Moore算法的核心思想是利用已知模式的信息,避免不必要的字符比较,提高搜索效率。对于您的需求,我们应该编写一个Boyer-Moore算法的版本来查找模式串`t`在目标串`s`中的位置。以下是简单的C语言实现示例:
```c
#include <stdio.h>
#include <string.h>
void BM_search(char *text, char *pattern) {
int n = strlen(text);
int m = strlen(pattern);
int i = m - 1; // pattern's ending position
while (i >= 0 && text[n - i] == pattern[i]) { // comparing the last character of pattern with the end of text
if (i == 0) { // found a match, print the starting index and break
printf("Pattern found at index %d\n", n - m);
return;
}
i--;
}
// Move to the right by the largest possible distance
for (; i >= 0; i--) {
while (text[n - i] != pattern[m - i - 1]) {
if (i == 0) {
printf("Pattern not found in the text\n");
return;
}
n -= m - i - 1;
}
if (n >= i + 1) {
printf("Pattern found at index %d\n", n - m);
return;
}
}
}
int main() {
char s[] = "abcabcabdabba";
char t[] = "abcabd";
BM_search(s, t);
return 0;
}
```
这个程序首先从字符串末尾开始逐个比较字符,如果找到匹配,则返回匹配位置;如果不匹配,则跳过较大的偏移量以减少无效比较。
阅读全文
相关推荐




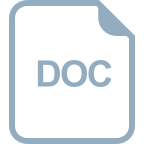
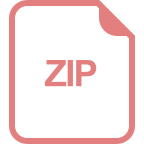
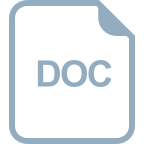


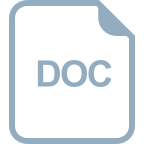








