ffmeg 硬件解码 demo
时间: 2024-01-18 12:00:53 浏览: 30
ffmpeg硬件解码demo主要是通过调用ffmpeg库中的接口来实现硬件加速解码功能。下面是一个简单的示例代码:
```c++
#include <stdio.h>
#include <stdbool.h>
#include <SDL2/SDL.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
int main(int argc, char* argv[]) {
// 初始化FFmpeg库
av_register_all();
avformat_network_init();
// 打开视频文件
AVFormatContext* formatCtx = avformat_alloc_context();
if (avformat_open_input(&formatCtx, argv[1], NULL, NULL) != 0) {
printf("无法打开输入文件\n");
return -1;
}
if (avformat_find_stream_info(formatCtx, NULL) < 0) {
printf("无法获取流信息\n");
return -1;
}
// 查找视频流
int videoStreamIndex = -1;
for (int i = 0; i < formatCtx->nb_streams; i++) {
if (formatCtx->streams[i]->codec->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
printf("无法找到视频流\n");
return -1;
}
// 获取解码器
AVCodec* codec = avcodec_find_decoder(formatCtx->streams[videoStreamIndex]->codecpar->codec_id);
if (codec == NULL) {
printf("找不到解码器\n");
return -1;
}
// 打开解码器
AVCodecContext* codecCtx = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codecCtx, formatCtx->streams[videoStreamIndex]->codecpar) != 0) {
printf("无法打开解码器\n");
return -1;
}
if (avcodec_open2(codecCtx, codec, NULL) < 0) {
printf("无法打开解码器\n");
return -1;
}
// 创建SDL窗口
SDL_Window* window = SDL_CreateWindow("FFmpeg硬件解码Demo", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, codecCtx->width, codecCtx->height, SDL_WINDOW_OPENGL);
if (window == NULL) {
printf("无法创建窗口\n");
return -1;
}
// 创建SDL渲染器
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, 0);
AVFrame* frame = av_frame_alloc();
AVPacket packet;
// 读取视频帧并渲染
bool quit = false;
while (!quit) {
if (av_read_frame(formatCtx, &packet) < 0) {
break;
}
if (packet.stream_index == videoStreamIndex) {
avcodec_send_packet(codecCtx, &packet);
while (avcodec_receive_frame(codecCtx, frame) == 0) {
SDL_RenderClear(renderer);
// 将帧数据复制到纹理
SDL_Texture* texture = SDL_CreateTexture(renderer, SDL_PIXELFORMAT_YV12, SDL_TEXTUREACCESS_STREAMING, codecCtx->width, codecCtx->height);
SDL_UpdateYUVTexture(texture, NULL, frame->data[0], frame->linesize[0], frame->data[1], frame->linesize[1], frame->data[2], frame->linesize[2]);
SDL_RenderCopy(renderer, texture, NULL, NULL);
SDL_RenderPresent(renderer);
SDL_DestroyTexture(texture);
SDL_Event event;
SDL_PollEvent(&event);
if (event.type == SDL_QUIT) {
quit = true;
break;
}
}
}
av_packet_unref(&packet);
}
// 释放资源
av_frame_free(&frame);
avcodec_close(codecCtx);
avformat_close_input(&formatCtx);
return 0;
}
```
这个示例代码使用了SDL库来进行窗口和渲染器的创建,通过SDL播放解码后的视频帧。其中,ffmpeg库提供了相关的接口方法来实现视频文件的打开、流信息的获取、解码器的查找和打开、帧的解码等功能。
相关推荐
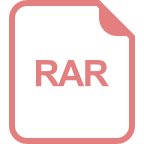














