定义一个复数类complex,利用重载实现复数的四则运算
时间: 2024-06-14 18:05:07 浏览: 203
以下是一个定义复数类Complex并利用运算符重载实现复数的四则运算的示例:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex() {
real = 0.0;
imag = 0.0;
}
Complex(double r) {
real = r;
imag = 0.0;
}
Complex(double r, double i) {
real = r;
imag = i;
}
Complex operator+(const Complex& c) {
Complex result;
result.real = real + c.real;
result.imag = imag + c.imag;
return result;
}
Complex operator-(const Complex& c) {
Complex result;
result.real = real - c.real;
result.imag = imag - c.imag;
return result;
}
Complex operator*(const Complex& c) {
Complex result;
result.real = real * c.real - imag * c.imag;
result.imag = real * c.imag + imag * c.real;
return result;
}
Complex operator/(const Complex& c) {
Complex result;
double denominator = c.real * c.real + c.imag * c.imag;
result.real = (real * c.real + imag * c.imag) / denominator;
result.imag = (imag * c.real - real * c.imag) / denominator;
return result;
}
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << " + " << c.imag << "i";
return os;
}
};
int main() {
Complex c1(2.0, 3.0);
Complex c2(1.0, 2.0);
Complex sum = c1 + c2;
Complex difference = c1 - c2;
Complex product = c1 * c2;
Complex quotient = c1 / c2;
cout << "Sum: " << sum << endl;
cout << "Difference: " << difference << endl;
cout << "Product: " << product << endl;
cout << "Quotient: " << quotient << endl;
return 0;
}
```
阅读全文
相关推荐
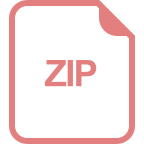
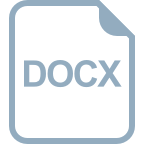
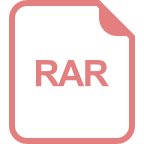










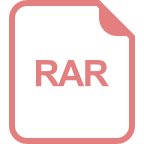
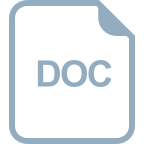
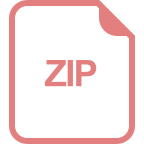
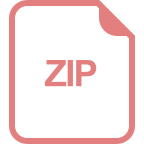
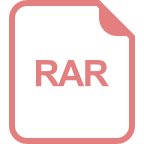
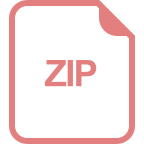