scanf("%d%s%s%d",&stu[i].number,&stu[i].name,&stu[i].sex,&stu[i].score);
时间: 2023-12-12 08:00:40 浏览: 68
&age, name, school, &grade); 是一行C语言的输入语句,可以从标准输入流中读取用户输入的数据。
其中"%d"是格式控制字符串,用于读取一个整数并将其存储在age变量中。"%s"是格式控制字符串,用于读取一个字符串并将其存储在name变量中。"%s"也是格式控制字符串,用于读取另一个字符串并将其存储在school变量中。"%d"是格式控制字符串,用于读取另一个整数并将其存储在grade变量中。
具体来说,这行代码意味着从标准输入流中依次读取用户输入的数据。首先会读取一个整数,这个整数会被存储在age变量中。然后会读取一个字符串,这个字符串会被存储在name变量中。接着会读取另一个字符串,这个字符串会被存储在school变量中。最后会读取最后一个整数,这个整数会被存储在grade变量中。
这行代码通常用于从用户获取输入并将输入的值存储在相应的变量中,以供后续的程序逻辑使用。然后程序可以根据这些变量的值进行相应的处理,比如根据年龄判断用户的年龄段,根据学校名称进行特定的操作,或者根据成绩进行评级等等。
需要注意的是,scanf函数的返回值为成功读取并赋值的参数个数,请在使用后检查返回值以确保输入的正确性。当输入的格式与代码中的格式字符串不匹配时,可能会导致错误的输入或结果。因此,在使用scanf函数时一定要谨慎并注意对各种可能的输入情况进行合理的处理和验证。
相关问题
struct student { int i; char name[10]; int chinese , math, english; }; int main(void) { int x, y; struct student stu; printf("input y:"); scanf("%d", y); printf("input the student's number,name and courage scores\n"); for (x = 1; x <= y; x++) { printf("No.%d:", x); scanf("%d%s%d%d%d", &stu.i, &stu.name, &stu.math, &stu.english, &stu.chinese); } printf("i:%d,name:%s,\n",stu.i,stu.name ); return 0; }
这段代码存在一些问题:
1. 在第6行中,应该使用取地址符&获取变量y的地址,即应该为scanf("%d", &y)。
2. 在第12行中,应该使用取地址符&获取结构体成员变量的地址,即应该为scanf("%d%s%d%d%d", &stu.i, stu.name, &stu.math, &stu.english, &stu.chinese)。
3. 在第14行中,应该输出循环中每个学生的成绩信息,而不是最后一个学生的信息。可以将printf语句放在循环中。
修改后的代码如下:
```
struct student {
int i;
char name[10];
int chinese, math, english;
};
int main(void) {
int x, y;
struct student stu;
printf("input y:");
scanf("%d", &y);
printf("input the student's number, name and course scores\n");
for (x = 1; x <= y; x++) {
printf("No.%d:", x);
scanf("%d%s%d%d%d", &stu.i, stu.name, &stu.math, &stu.english, &stu.chinese);
printf("No.%d: i:%d, name:%s, math:%d, english:%d, chinese:%d\n", x, stu.i, stu.name, stu.math, stu.english, stu.chinese);
}
return 0;
}
```
#include<stdio.h> struct student { *学生信息结构定义* int num; 冰学号* char name[10]; 冰姓名* intcomputer,english,math;1*三门课程成繢*/ double average; *个人平均成绩*/ }; int main() int i, n; struct student stu,max; 冰定义结构变量* printf ("Input n: "); scanf ("%d", &n); printf ("Input the student's number, name and course scores\n"); for (i = 1; i <= n; i++){ printf ("No.%d: ", i); scanf %d".&stu.num,stu.name,&stu.math,&stu.english,&stu.computer); stu.average = (stu.math + stu.english + stu.computer) / 3.0; if (i == 1){ max = stu; 冰结构变量赋值*/ Felseif(max.average<stu.average){ max = stu; ["%d%5%d%d 冰结构变量赋值*/ Felseif(max.average<stu.average){ max = stu; ["%d%5%d%d printf("num:%d, max.average); return 0; name:%5, average:%.2Ifn", max.num, max.name, ) return 0; }向这个代码里面添加查询和修改功能
要添加查询和修改功能,需要在主函数中添加相应的代码。下面是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
struct student {
int num; // 学号
char name[10]; // 姓名
int computer; // 计算机成绩
int english; // 英语成绩
int math; // 数学成绩
double average; // 平均成绩
};
int main() {
int i, n, choice, num;
struct student stu, max = {0};
printf("Input n: ");
scanf("%d", &n);
printf("Input the student's number, name and course scores\n");
for (i = 1; i <= n; i++) {
printf("No.%d: ", i);
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
if (i == 1) {
max = stu;
} else if (max.average < stu.average) {
max = stu;
}
}
printf("num:%d, name:%s, average:%.2f\n", max.num, max.name, max.average);
// 添加查询和修改功能
printf("Enter 1 to query a student's information, 2 to modify a student's information: ");
scanf("%d", &choice);
if (choice == 1) {
printf("Enter the student's number: ");
scanf("%d", &num);
for (i = 1; i <= n; i++) {
printf("No.%d: ", i);
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
if (stu.num == num) {
printf("num:%d, name:%s, math:%d, english:%d, computer:%d, average:%.2f\n", stu.num, stu.name, stu.math, stu.english, stu.computer, stu.average);
break;
}
}
if (i > n) {
printf("Student not found.\n");
}
} else if (choice == 2) {
printf("Enter the student's number: ");
scanf("%d", &num);
for (i = 1; i <= n; i++) {
printf("No.%d: ", i);
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
if (stu.num == num) {
printf("Enter the new information:\n");
scanf("%d%s%d%d%d", &stu.num, stu.name, &stu.math, &stu.english, &stu.computer);
stu.average = (stu.math + stu.english + stu.computer) / 3.0;
printf("num:%d, name:%s, math:%d, english:%d, computer:%d, average:%.2f\n", stu.num, stu.name, stu.math, stu.english, stu.computer, stu.average);
break;
}
}
if (i > n) {
printf("Student not found.\n");
}
} else {
printf("Invalid choice.\n");
}
return 0;
}
```
在上面的代码中,我们添加了一个 `choice` 变量,用于选择查询或修改功能。当用户选择查询时,我们需要再次输入要查询的学生的学号,然后遍历学生数组,找到对应学号的学生,输出其信息。当用户选择修改时,我们也需要输入要修改的学生的学号,然后遍历学生数组,找到对应学号的学生,再次输入该学生的信息,更新结构体中的信息。如果找不到对应学号的学生,输出相应的错误信息。
阅读全文
相关推荐
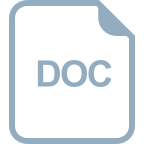
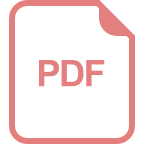













