用C语言编写程序:通过键盘读取元素建立一个线性表;指定一个元素,在此元素之前插入一个新元素;指定一个元素,删除此元素。·
时间: 2024-10-14 07:18:29 浏览: 28
在C语言中,我们可以使用数组、结构体以及指针来创建简单的线性表,并实现插入和删除操作。这里是一个基础示例,我们将使用动态内存分配创建线性表并实现功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义线性表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建线性表
Node* createList() {
int input;
printf("请输入元素的数量:");
scanf("%d", &input);
Node* head = (Node*)malloc(sizeof(Node)); // 动态分配头结点
if (!head) {
printf("内存分配失败!");
return NULL;
}
head->data = 0; // 设置默认值
head->next = NULL;
Node* current = head;
for (int i = 1; i < input; ++i) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
free(current); // 如果前面分配失败,则释放当前节点
return NULL;
}
printf("请输入第 %d 个元素:", i+1);
scanf("%d", &newNode->data);
newNode->next = current->next;
current->next = newNode;
current = newNode;
}
return head;
}
// 在指定元素前插入新元素
void insertBefore(Node** head, int target, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("内存分配失败!");
return;
}
newNode->data = value;
Node* current = *head;
while (current != NULL && current->data != target) {
current = current->next;
}
if (current == NULL) {
printf("目标元素未找到!");
return;
}
newNode->next = current->next;
current->next = newNode;
}
// 删除指定元素
void deleteElement(Node** head, int target) {
Node* current = *head;
Node* previous = NULL;
if (current != NULL && current->data == target) {
*head = current->next;
free(current);
return;
}
while (current != NULL && current->data != target) {
previous = current;
current = current->next;
}
if (current == NULL) {
printf("目标元素未找到!");
return;
}
previous->next = current->next;
free(current);
}
int main() {
Node* list = createList();
if (list != NULL) {
int action;
printf("\n请选择操作:1 插入 2 删除\n");
scanf("%d", &action);
switch (action) {
case 1:
int position, new_value;
printf("请输入要在哪一位元素前插入:");
scanf("%d", &position);
printf("请输入新插入的值:");
scanf("%d", &new_value);
insertBefore(&list, position, new_value);
break;
case 2:
int target_to_delete;
printf("请输入要删除的元素:");
scanf("%d", &target_to_delete);
deleteElement(&list, target_to_delete);
break;
default:
printf("无效的操作选择!");
break;
}
}
return 0;
}
```
阅读全文
相关推荐
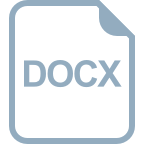
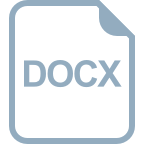
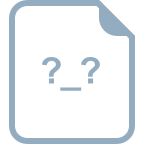















