通过键盘读取元素建立一个线性表;指定一个元素,在此元素之前插入一个新元素;指定一个元素,删除此元素。用c实现上述内容
时间: 2024-10-11 20:08:35 浏览: 7
在C语言中,你可以创建一个链表数据结构来满足上述需求,因为链表可以动态地添加和删除节点。这里我们首先定义一个链表节点结构,然后实现插入和删除操作。由于题目中提到数组,这里先用数组作为例子演示如何在特定位置插入和删除元素,后续再转换为链表。
**数组版本实现:**
```c
#include <stdio.h>
#include <stdlib.h>
#define ARRAY_SIZE 10
typedef struct {
int data;
int index; // 用于记录元素在数组中的位置
} Node;
Node array[ARRAY_SIZE];
int list_size = 0;
// 插入元素
void insertBefore(int target, int new_data) {
for (int i = 0; i < list_size; i++) {
if (array[i].data == target) {
if (i + 1 < ARRAY_SIZE) { // 在目标元素之后有空间
array[i+1].data = new_data;
array[i].index++; // 更新目标元素索引
} else {
printf("Array full, cannot insert before %d\n", target);
}
break;
}
}
if (new_data != -1) list_size++; // 只有新的有效数据才增加大小
}
// 删除元素
void removeElement(int target) {
for (int i = 0; i < list_size; i++) {
if (array[i].data == target) {
memmove(&array[i], &array[i+1], sizeof(Node)*(list_size-i-1)); // 移动元素
list_size--; // 减小数组大小
break;
}
}
}
int main() {
while (true) {
int choice;
printf("1. Insert before a specific element\n2. Remove an element\n3. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
int target, new_data;
printf("Enter the target element and then the new data: ");
scanf("%d %d", &target, &new_data);
insertBefore(target, new_data);
break;
case 2:
int delete_target;
printf("Enter the element to delete: ");
scanf("%d", &delete_target);
removeElement(delete_target);
break;
case 3:
return 0;
default:
printf("Invalid choice, try again.\n");
}
}
}
```
**
相关推荐
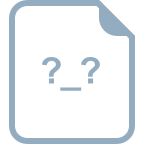
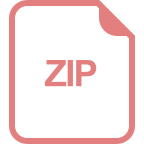
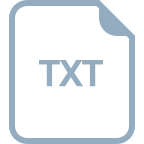










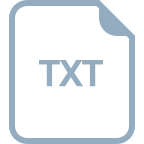
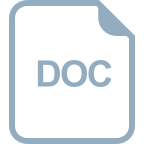
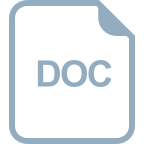
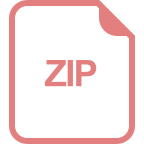
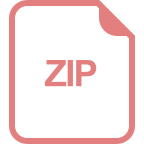