通过键盘读取元素建立一个线性表;指定一个元素,在此元素之前插入一个新元素;指定一个元素,删除此元素。用c语言实现
时间: 2024-10-11 10:08:15 浏览: 29
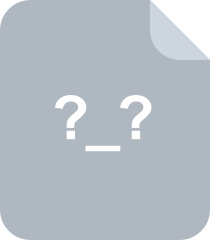
有一个线性表(a1,a2,...,an),它存储在有附加表头结点的单链表中,写一个算法,求出该线性表中值为x的元素的序号。如果x

在 C 语言中,我们可以使用数组来实现简单的线性表。为了满足题目描述的操作,我们通常会维护一个动态大小的数组,并提供相应的函数来进行插入和删除操作。这里是一个基础的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 定义结构体表示线性表节点
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化线性表,添加第一个元素
void init_list(Node** head) {
*head = (Node*)malloc(sizeof(Node));
if (*head == NULL) {
printf("Memory allocation failed.\n");
return;
}
(*head)->data = -1; // 空表标记
(*head)->next = NULL;
}
// 在指定位置插入新元素
void insert_before(Node** head, int value, int position) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head;
for (int i = 0; i < position - 1 && *head != NULL; i++) {
*head = (*head)->next;
}
if (*head != NULL) {
(*head)->next = newNode;
} else {
printf("Invalid insertion position.\n");
}
}
// 删除指定元素
void delete_node(Node** head, int value) {
if (*head == NULL) {
return;
}
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = temp->next;
free(temp);
} else {
printf("Element not found in the list.\n");
}
}
// 打印列表
void print_list(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
init_list(&head);
// 示例操作
insert_before(&head, 5, 0); // 在头部插入 5
insert_before(&head, 4, 1); // 在第二个位置(索引1)插入 4
print_list(head); // 打印列表
delete_node(&head, 4); // 删除值为 4 的元素
print_list(head); // 再次打印列表
return 0;
}
```
阅读全文
相关推荐
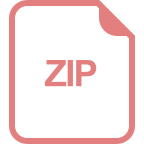
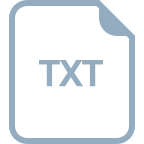












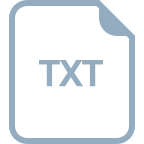
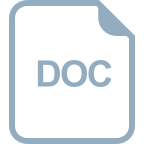
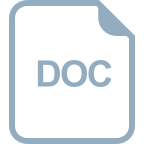
