通过键盘读取元素建立一个线性表;指定一个元素,在此元素之前插入一个新元素;指定一个元素,删除此元素。用一串c语言代码实现以上内容
时间: 2024-10-11 20:08:36 浏览: 29
在C语言中,我们可以使用结构体来表示线性表,并提供相应的插入和删除操作。这里是一个简单的例子,我们首先创建一个结构体`Node`表示列表节点,然后实现一个`List`类来管理这个线性表。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建链表
struct List {
Node* head;
};
// 插入元素函数
void insertBefore(List* list, int target, int new_data) {
// 找到目标元素的前一个节点
Node* prev = NULL;
Node* current = list->head;
while (current != NULL && current->data != target) {
prev = current;
current = current->next;
}
// 如果找到目标,插入新节点
if (prev != NULL) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = new_data;
newNode->next = prev->next;
prev->next = newNode;
} else {
printf("Element not found.\n");
}
}
// 删除元素函数
void deleteElement(List* list, int target) {
// 遍历查找目标节点
Node* current = list->head;
Node* previous = NULL;
while (current != NULL) {
if (current->data == target) {
// 如果找到了,删除当前节点
if (previous == NULL) { // 作为头结点的情况
list->head = current->next;
} else {
previous->next = current->next;
}
free(current); // 释放内存
return; // 结束删除操作
}
previous = current;
current = current->next;
}
printf("Element not found.\n");
}
// 主函数示例
int main() {
List list;
list.head = NULL;
// 假设已经有一些初始数据插入
// ... (实际代码中添加元素)
// 插入示例
insertBefore(&list, 50, 60);
// 删除示例
deleteElement(&list, 60);
return 0;
}
阅读全文
相关推荐
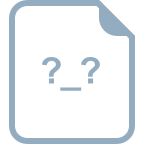
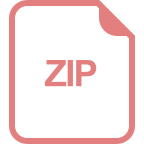
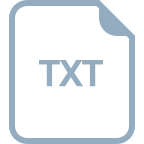












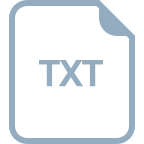
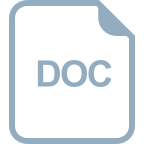
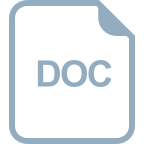
