编写程序,实现二叉树的前序遍历,中序遍历,后序遍历
时间: 2024-06-29 21:01:10 浏览: 104
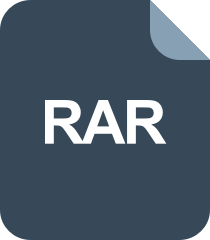
实现先序,中序和后序遍历的二叉树遍历程序

在编程中,实现二叉树的遍历主要有三种方式:前序遍历(Preorder Traversal)、中序遍历(Inorder Traversal)和后序遍历(Postorder Traversal)。这些遍历都是深度优先搜索(Depth-First Search, DFS)的一种体现,但访问节点的顺序不同。
**1. 前序遍历(Preorder):**
- 首先访问根节点,
- 然后递归地遍历左子树,
- 最后遍历右子树。
**示例代码(递归实现):**
```python
def preorder_traversal(root):
if root is not None:
print(root.val) # 访问根节点
preorder_traversal(root.left) # 遍历左子树
preorder_traversal(root.right) # 遍历右子树
```
**2. 中序遍历(Inorder):**
- 首先递归地遍历左子树,
- 然后访问根节点,
- 最后遍历右子树。
**示例代码(递归实现):**
```python
def inorder_traversal(root):
if root is not None:
inorder_traversal(root.left) # 遍历左子树
print(root.val) # 访问根节点
inorder_traversal(root.right) # 遍历右子树
```
**3. 后序遍历(Postorder):**
- 首先递归地遍历左子树,
- 然后递归地遍历右子树,
- 最后访问根节点。
**示例代码(递归实现):**
```python
def postorder_traversal(root):
if root is not None:
postorder_traversal(root.left) # 遍历左子树
postorder_traversal(root.right) # 遍历右子树
print(root.val) # 访问根节点
```
阅读全文
相关推荐
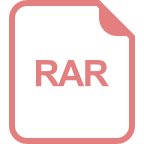
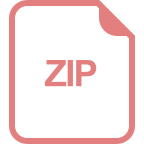






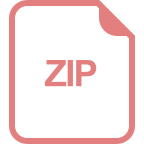
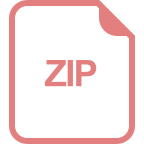
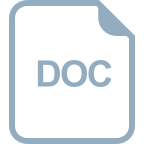




