正规文法与有限自动机相互转换c++代码实现
时间: 2024-06-12 18:10:41 浏览: 210
好的,这是一个比较复杂的问题,需要一定的计算机理论基础。我会尽可能简单地解释一下。
正规文法与有限自动机是两种描述形式语言的方式。正规文法是一种用于描述正则语言的形式化语法,而有限自动机是一种用于识别正则语言的自动机。
正规文法可以转换为有限自动机,这个过程叫做正规文法到NFA的转换。具体步骤如下:
1. 将正规文法转换为正则表达式。
2. 将正则表达式转换为NFA。
下面是一个简单的C++代码实现:
```c++
#include <iostream>
#include <stack>
#include <vector>
#include <string>
using namespace std;
// 定义状态节点
struct State {
int id;
bool is_final;
vector<pair<char, State*>> transitions;
};
// 定义NFA
class NFA {
public:
NFA(State* start, State* end) : start(start), end(end) {}
State* start;
State* end;
};
// 定义正则表达式节点
struct RegexNode {
char c;
RegexNode* left;
RegexNode* right;
RegexNode(char c) : c(c), left(nullptr), right(nullptr) {}
RegexNode(char c, RegexNode* left, RegexNode* right) : c(c), left(left), right(right) {}
};
// 将正规文法转换为正则表达式
RegexNode* grammarToRegex(string grammar) {
stack<RegexNode*> s;
for (char c : grammar) {
if (c == '*') {
RegexNode* node = s.top();
s.pop();
s.push(new RegexNode(c, node, nullptr));
} else if (c == '|') {
RegexNode* right = s.top();
s.pop();
RegexNode* left = s.top();
s.pop();
s.push(new RegexNode(c, left, right));
} else if (c == '.') {
RegexNode* right = s.top(); s.pop();
RegexNode* left = s.top();
s.pop();
s.push(new RegexNode(c, left, right));
} else {
s.push(new RegexNode(c));
}
}
return s.top();
}
// 将正则表达式转换为NFA
NFA regexToNFA(RegexNode* node, int& state_id) {
if (node->c == '*') {
NFA nfa = regexToNFA(node->left, state_id);
State* new_start = new State{state_id++, false, {{' ', nfa.start}, {' ', nullptr}}};
State* new_end = new State{state_id++, true, {{' ', nfa.start}, {' ', nullptr}}};
nfa.end->transitions.push_back({' ', nfa.start});
nfa.end->transitions.push_back({' ', new_end});
new_start->transitions.push_back({' ', nfa.start});
new_start->transitions.push_back({' ', new_end});
return NFA(new_start, new_end);
} else if (node->c == '|') {
NFA left_nfa = regexToNFA(node->left, state_id);
NFA right_nfa = regexToNFA(node->right, state_id);
State* new_start = new State{state_id++, false, {{' ', left_nfa.start}, {' ', right_nfa.start}}};
State* new_end = new State{state_id++, true, {}};
left_nfa.end->transitions.push_back({' ', new_end});
right_nfa.end->transitions.push_back({' ', new_end});
return NFA(new_start, new_end);
} else if (node->c == '.') {
NFA left_nfa = regexToNFA(node->left, state_id);
NFA right_nfa = regexToNFA(node->right, state_id);
left_nfa.end->transitions.push_back({' ', right_nfa.start});
return NFA(left_nfa.start, right_nfa.end);
} else {
State* start = new State{state_id++, false, {}};
State* end = new State{state_id++, true, {}};
start->transitions.push_back({node->c, end});
return NFA(start, end);
}
}
int main() {
string grammar = "a.b|c*";
RegexNode* regex = grammarToRegex(grammar);
int state_id = 0;
NFA nfa = regexToNFA(regex, state_id);
// 输出NFA
cout << "NFA:\n";
cout << "Start state: " << nfa.start->id << "\n";
cout << "End state: " << nfa.end->id << "\n";
for (State* state : {nfa.start, nfa.end}) {
cout << "State " << state->id << ": ";
if (state->is_final) {
cout << "final\n";
} else {
cout << "not final\n";
}
for (auto transition : state->transitions) {
cout << " " << transition.first << " -> " << transition.second->id << "\n";
}
}
return 0;
}
```
阅读全文
相关推荐
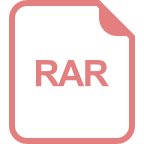
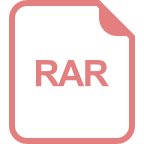

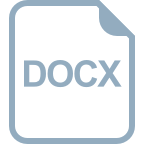
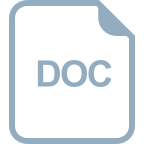
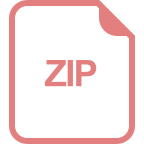
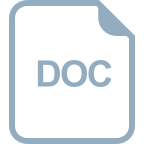
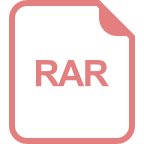
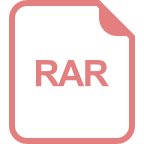
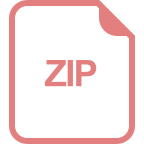
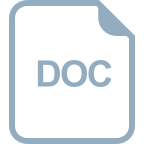
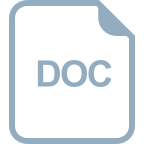
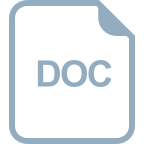
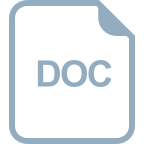
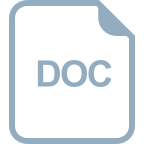
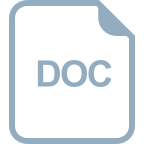
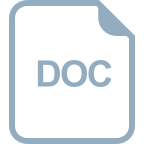
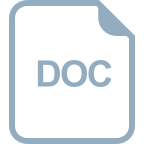