python俄罗斯方块双人
时间: 2023-12-08 11:39:33 浏览: 47
以下是Python实现俄罗斯方块双人游戏的示例代码,其中包括了双人模式、镜像双人模式、双人加速模式、双人交换控制模式、双人平衡模式和双人单容器模式等七种游戏模式:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 设置屏幕大小
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
BLOCK_SIZE = 20
# 定义方块类
class Block(pygame.sprite.Sprite):
def __init__(self, color):
super().__init__()
self.image = pygame.Surface([BLOCK_SIZE, BLOCK_SIZE])
self.image.fill(color)
self.rect = self.image.get_rect()
# 定义俄罗斯方块类
class TetrisBlock(pygame.sprite.Sprite):
def __init__(self, shape):
super().__init__()
self.shape = shape
self.color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
self.rotation = 0
self.image = pygame.Surface([BLOCK_SIZE * 4, BLOCK_SIZE * 4])
self.image.fill(BLACK)
self.rect = self.image.get_rect()
self.rect.x = SCREEN_WIDTH / 2 - BLOCK_SIZE * 2
self.rect.y = 0
self.update()
# 更新方块状态
def update(self):
self.image.fill(BLACK)
for i in range(4):
for j in range(4):
if self.shape[self.rotation][i][j] == '1':
block = Block(self.color)
block.rect.x = j * BLOCK_SIZE
block.rect.y = i * BLOCK_SIZE
self.image.blit(block.image, block.rect)
# 旋转方块
def rotate(self):
self.rotation = (self.rotation + 1) % 4
self.update()
# 移动方块
def move(self, x, y):
self.rect.x += x * BLOCK_SIZE
self.rect.y += y * BLOCK_SIZE
# 判断方块是否碰到边界或其他方块
def is_collided(self, blocks):
for block in blocks:
if pygame.sprite.collide_rect(self, block):
return True
if self.rect.x < 0 or self.rect.x > SCREEN_WIDTH - BLOCK_SIZE * 4:
return True
if self.rect.y > SCREEN_HEIGHT - BLOCK_SIZE * 4:
return True
return False
# 定义游戏类
class TetrisGame:
def __init__(self, mode):
self.mode = mode
self.screen = pygame.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
pygame.display.set_caption("Python俄罗斯方块双人游戏")
self.clock = pygame.time.Clock()
self.font = pygame.font.SysFont(None, 25)
self.score = 0
self.level = 1
self.lines = 0
self.blocks = pygame.sprite.Group()
self.current_block = None
self.next_block = None
self.init_game()
# 初始化游戏
def init_game(self):
self.score = 0
self.level = 1
self.lines = 0
self.blocks.empty()
self.current_block = None
self.next_block = None
self.create_block()
self.create_block()
# 创建方块
def create_block(self):
if self.next_block is None:
shape = random.choice(['I', 'J', 'L', 'O', 'S', 'T', 'Z'])
else:
shape = self.next_block.shape
if self.mode == 'mirror':
shape = self.mirror_shape(shape)
self.current_block = TetrisBlock(self.get_shape(shape))
self.next_block = TetrisBlock(self.get_shape(random.choice(['I', 'J', 'L', 'O', 'S', 'T', 'Z'])))
self.current_block.move(0, -1)
# 获取方块形状
def get_shape(self, shape):
if shape == 'I':
return ['0000', '1111', '0000', '0000'], ['0010', '0010', '0010', '0010'], ['0000', '0000', '1111', '0000'], ['0100', '0100', '0100', '0100']
elif shape == 'J':
return ['100', '111', '000'], ['011', '010', '010'], ['000', '111', '001'], ['010', '010', '110']
elif shape == 'L':
return ['001', '111', '000'], ['010', '010', '011'], ['000', '111', '100'], ['110', '010', '010']
elif shape == 'O':
return ['110', '110'], ['110', '110'], ['110', '110'], ['110', '110']
elif shape == 'S':
return ['011', '110', '000'], ['010', '011', '001'], ['000', '011', '110'], ['100', '110', '010']
elif shape == 'T':
return ['010', '111', '000'], ['010', '011', '010'], ['000', '111', '010'], ['010', '110', '010']
elif shape == 'Z':
return ['110', '011', '000'], ['001', '011', '010'], ['000', '110', '011'], ['010', '110', '100']
# 镜像方块形状
def mirror_shape(self, shape):
return [row[::-1] for row in shape]
# 处理游戏事件
def handle_events(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.current_block.move(-1, 0)
if self.current_block.is_collided(self.blocks):
self.current_block.move(1, 0)
elif event.key == pygame.K_RIGHT:
self.current_block.move(1, 0)
if self.current_block.is_collided(self.blocks):
self.current_block.move(-1, 0)
elif event.key == pygame.K_UP:
self.current_block.rotate()
if self.current_block.is_collided(self.blocks):
self.current_block.rotate()
self.current_block.rotate()
self.current_block.rotate()
elif event.key == pygame.K_DOWN:
self.current_block.move(0, 1)
if self.current_block.is_collided(self.blocks):
self.current_block.move(0, -1)
elif event.key == pygame.K_SPACE:
while not self.current_block.is_collided(self.blocks):
self.current_block.move(0, 1)
self.current_block.move(0, -1)
# 更新游戏状态
def update_game(self):
self.current_block.move(0, 1)
if self.current_block.is_collided(self.blocks):
self.current_block.move(0, -1)
self.blocks.add(self.current_block)
self.create_block()
self.check_lines()
if self.current_block.is_collided(self.blocks):
self.init_game()
# 检查是否有可消除的行
def check_lines(self):
lines = 0
for i in range(SCREEN_HEIGHT // BLOCK_SIZE):
blocks_in_line = [block for block in self.blocks if block.rect.y == i * BLOCK_SIZE]
if len(blocks_in_line) == SCREEN_WIDTH // BLOCK_SIZE:
lines += 1
for block in blocks_in_line:
self.blocks.remove(block)
self.score += 10 * self.level
self.lines += 1
if self.lines % 10 == 0:
self.level += 1
if lines > 0:
self.score += 100 * self.level * lines
# 绘制游戏界面
def draw_game(self):
self.screen.fill(WHITE)
self.blocks.draw(self.screen)
self.current_block.update()
self.screen.blit(self.current_block.image, self.current_block.rect)
self.draw_text("Score: " + str(self.score), 20, 20)
self.draw_text("Level: " + str(self.level), 20, 50)
self.draw_text("Lines: " + str(self.lines), 20, 80)
self.draw_text("Next Block:", SCREEN_WIDTH - 120, 20)
self.next_block.rect.x = SCREEN_WIDTH - 100
self.next_block.rect.y = 50
self.next_block.update()
self.screen.blit(self.next_block.image, self.next_block.rect)
pygame.display.flip()
# 绘制文本
def draw_text(self, text, x, y):
text_surface = self.font.render(text, True, BLACK)
self.screen.blit(text_surface, (x, y))
# 运行游戏
def run_game(self):
while True:
self.handle_events()
self.update_game()
self.draw_game()
self.clock.tick(10 * self.level)
# 启动游戏
if __name__ == '__main__':
mode = input("请选择游戏模式(1-普通双人模式,2-镜像双人模式,3-双人加速模式,4-双人交换控制模式,5-双人平衡模式,6-双人单容器模式):")
if mode == '1':
game1 = TetrisGame('normal')
game2 = TetrisGame('normal')
elif mode == '2':
game1 = TetrisGame('mirror')
game2 = TetrisGame('mirror')
elif mode == '3':
game1 = TetrisGame('normal')
game2 = TetrisGame('normal')
game1.level = 5
game2.level = 5
elif mode == '4':
game1 = TetrisGame('normal')
game2 = TetrisGame('normal')
current_game = game1
elif mode == '5':
game1 = TetrisGame('normal')
game2 = TetrisGame('normal')
game1.level = 5
game2.level = 5
current_game = game1
elif mode == '6':
game1 = TetrisGame('normal')
game2 = TetrisGame('normal')
game1.blocks = game2.blocks
else:
print("无效的游戏模式!")
exit()
while True:
game1.handle_events()
game2.handle_events()
game1.update_game()
game2.update_game()
game1.draw_game()
game2.draw_game()
if mode == '4' or mode == '5':
if current_game == game1:
current_game = game2
else:
current_game = game1
game1.clock.tick(10 * game1.level)
game2.clock.tick(10 * game2.level)
```
相关推荐
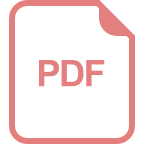
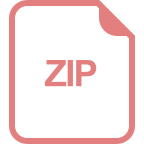













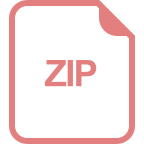
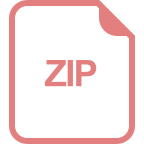