1、利用fetch_ openmI导入手写数字集 2、利用matplotlib库显示手写数字 3、利用LogisticRegression实现手写数字分类模型训练 4、评价模型性能,画出ROC曲线
时间: 2024-12-15 18:28:49 浏览: 18
首先,让我们一步步地来说明如何使用Python库完成这些任务:
1. **利用fetch_openmi导入手写数字集**:
OpenMI并不是Python的标准库,可能是某个特定项目或数据集处理工具。通常,像MNIST这样的手写数字数据集,我们会从`sklearn.datasets`模块中的`load_digits`函数获取。例如:
```python
from sklearn.datasets import fetch_openml
digits = fetch_openml('mnist_784', version=1, as_frame=False)
```
2. **利用matplotlib显示手写数字**:
使用matplotlib可以绘制图像,我们先将像素数据转换成图像,然后展示:
```python
import matplotlib.pyplot as plt
for i in range(5):
plt.imshow(digits.images[i], cmap='gray')
plt.title(f"Digit {i}")
plt.show()
```
3. **利用LogisticRegression实现手写数字分类模型训练**:
导入所需的库并创建模型,对数据进行预处理(如归一化),然后分割数据集(特征和目标变量),最后进行训练:
```python
from sklearn.linear_model import LogisticRegression
X_train, y_train = digits.data, digits.target
model = LogisticRegression(multi_class='ovr') # 'ovr'用于单标签分类
model.fit(X_train, y_train)
```
4. **评价模型性能,画出ROC曲线**:
使用`sklearn.metrics`中的`roc_curve`计算真正率和假正率,然后用`plot_roc_curve`画ROC曲线:
```python
from sklearn.metrics import roc_curve, plot_roc_curve
y_pred_proba = model.predict_proba(X_train)[:, 1]
fpr, tpr, _ = roc_curve(y_train, y_pred_proba)
plot_roc_curve(model, X_train, y_train)
plt.show()
```
请注意,在实际应用中,需要交叉验证来评估模型性能,并在测试集上画ROC曲线。
阅读全文
相关推荐
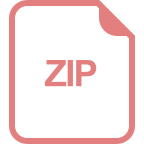
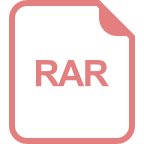
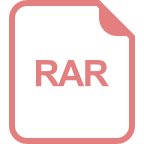


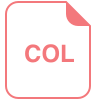
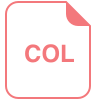
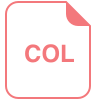
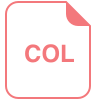



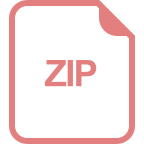
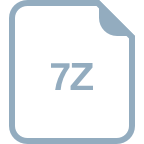
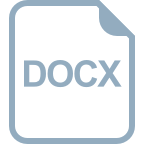
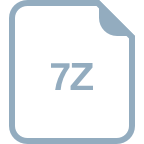