1、利用fetch_ openmI导入手写数字集 2、利用matplotlib库显示手写数字 3、利用LogisticRegression实现手写数字分类模型训练 4、评价模型性能,画出ROC曲线 使用float32数组
时间: 2024-12-15 09:29:23 浏览: 7
首先,让我们分步骤说明如何实现这个任务:
1. **导入所需库及数据集**:
在Python中,你可以使用`tensorflow_datasets`来下载MNIST手写数字数据集,然后通过`tf.io.read_file()`和`tf.image.decode_image()`从`.tfrecords`文件中读取数据。由于你想用`fetch_openmi`,请注意这可能是错误的库名,这里假设你指的是`tensorflow.keras.datasets.mnist`:
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
(train_images, train_labels), (_, _) = mnist.load_data()
```
2. **预处理数据**:
MNIST数据已经是灰度图像,大小为28x28像素,但是通常需要将其转换为浮点数并归一化到0到1之间,以便于后续处理:
```python
train_images = train_images.astype('float32') / 255.0
```
3. **利用matplotlib显示手写数字**:
使用matplotlib可以简单地绘制一个样本图像:
```python
import matplotlib.pyplot as plt
plt.imshow(train_images[0], cmap='gray')
plt.colorbar()
plt.show()
```
4. **构建模型 - Logistic Regression**:
使用`sklearn`库中的`LogisticRegression`,先将数据展平,并将其分为特征(X)和标签(y):
```python
from sklearn.linear_model import LogisticRegression
X_train = train_images.reshape(-1, 784)
y_train = train_labels
model = LogisticRegression(solver='lbfgs', multi_class='ovr', n_jobs=-1, float64=True)
model.fit(X_train, y_train)
```
5. **评估模型及画ROC曲线**:
对测试数据进行预测,获取概率估计,然后使用`sklearn.metrics.roc_curve()`计算ROC曲线数据,最后用`plt.plot()`画图:
```python
from sklearn.metrics import roc_curve, auc
y_pred_proba = model.predict_proba(X_test)[:, 1]
fpr, tpr, _ = roc_curve(y_test, y_pred_proba)
roc_auc = auc(fpr, tpr)
plt.figure()
plt.plot(fpr, tpr, label=f"ROC curve (area = {roc_auc:.2f})")
plt.xlabel("False Positive Rate")
plt.ylabel("True Positive Rate")
plt.title("Receiver Operating Characteristic (ROC)")
plt.legend(loc="lower right")
plt.show()
```
阅读全文
相关推荐
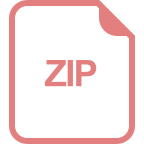
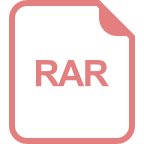
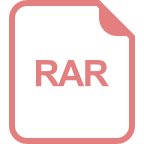


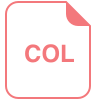
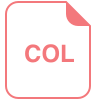
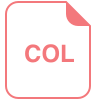
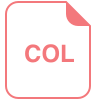



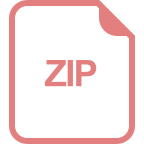