python正则表达式提取字符串中汉字
时间: 2023-07-31 11:06:06 浏览: 138
要使用正则表达式提取字符串中的汉字,你可以使用 Python 的 re 模块。以下是一个示例代码,演示如何提取字符串中的汉字:
```python
import re
# 定义一个包含汉字的字符串
string = "这是一个包含汉字的字符串"
# 使用正则表达式提取所有汉字
chinese_chars = re.findall(r'[\u4e00-\u9fff]+', string)
# 打印提取到的汉字
print(chinese_chars)
```
在上面的示例中,使用 `re.findall()` 函数和正则表达式 `[\u4e00-\u9fff]+` 来提取字符串中的汉字。该正则表达式表示匹配一个或多个连续的汉字。`[\u4e00-\u9fff]` 是 Unicode 编码范围,表示汉字的范围。
运行上述代码,输出将会是 `['这是一个包含汉字的字符串']`,即从字符串中提取到的汉字。
如果你想提取其他形式的汉字,例如只提取单个汉字,可以根据具体需求修改正则表达式。
希望对你有所帮助!如有其他问题,请随时提问。
相关问题
python正则表达式提取字符串人物名字
可以使用以下正则表达式来提取字符串中的人物名字:
```
import re
text = "王小明、李大雄和张三丰是三个人物。"
pattern = r"[\u4e00-\u9fa5]+"
matches = re.findall(pattern, text)
for match in matches:
print(match)
```
输出结果:
```
王小明
李大雄
张三丰
```
解释:
- `[\u4e00-\u9fa5]` 表示中文字符的范围,即 Unicode 编码中的中文字符。
- `+` 表示匹配一个或多个前面的字符。
- `re.findall(pattern, text)` 表示在 `text` 中查找所有符合 `pattern` 的字符串,并返回一个列表。
python 读取用正则表达式读取字符串中的中文
可以使用re模块中的正则表达式匹配中文字符。
以下是一个例子,假设要提取字符串中的中文:
```python
import re
string = "Hello, 你好,世界!"
# 匹配中文字符
pattern = re.compile(r'[\u4e00-\u9fa5]+')
# 提取中文字符
result = pattern.findall(string)
# 输出结果
print(result) # ['你好', '世界']
```
其中,正则表达式`[\u4e00-\u9fa5]+`表示匹配一个或多个中文字符。`findall`方法可以返回所有匹配的结果。
相关推荐
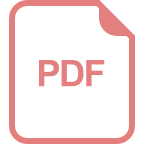
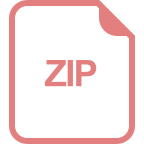
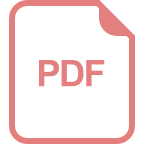












