C语言 串口设置 8N1 无流控 无校验
时间: 2023-09-19 08:10:51 浏览: 72
在 C 语言中设置串口为 8N1,无流控和无校验,可以使用下面的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int set_interface_attribs(int fd, int speed, int parity)
{
struct termios tty;
memset(&tty, 0, sizeof(tty));
if (tcgetattr(fd, &tty) != 0) {
printf("error %d from tcgetattr\n", errno);
return -1;
}
cfsetospeed(&tty, speed);
cfsetispeed(&tty, speed);
// 8N1
tty.c_cflag &= ~PARENB;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8;
// 无流控
tty.c_cflag &= ~CRTSCTS;
// 无校验
tty.c_cflag &= ~PARODD;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CREAD | CLOCAL; // turn on READ & ignore ctrl lines
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // turn off s/w flow ctrl
tty.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // make raw
tty.c_cc[VMIN] = 0;
tty.c_cc[VTIME] = 5;
tcsetattr(fd, TCSANOW, &tty);
return 0;
}
int main()
{
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_SYNC);
if (fd < 0) {
printf("error %d opening /dev/ttyS0: %s\n", errno, strerror(errno));
return -1;
}
set_interface_attribs(fd, B9600, 0); // set speed to 9600 bps, 8N1 (no parity)
return 0;
}
```
其中,set_interface_attribs 函数用于设置串口参数,fd 参数为串口文件描述符,speed 参数为波特率,parity 参数为校验位类型(0 表示无校验位,其他值表示有校验位)。在函数中,首先调用 tcgetattr 函数获取当前串口参数,然后根据需要的参数值进行设置,最后调用 tcsetattr 函数将参数设置应用到串口。在主函数中,打开串口文件,然后调用 set_interface_attribs 函数设置串口参数即可。
相关推荐
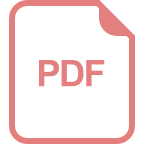














