x = np.array(list(range(1, 11))).reshape(-1, 1) 什么意思详细解释一下
时间: 2023-09-02 21:07:18 浏览: 218
这行代码使用了NumPy库,定义了一个一维数组,该数组由1到10的数字构成。然后使用reshape方法将该数组转换为一个列向量(二维数组),即将原数组的行数变为1,列数变为10,该操作使用了参数-1,表示该维度的长度应该自动计算得出,以保证总元素个数不变。最终得到的数组x如下所示:
```
array([[ 1],
[ 2],
[ 3],
[ 4],
[ 5],
[ 6],
[ 7],
[ 8],
[ 9],
[10]])
```
可以使用print(x.shape)查看数组的形状,输出结果为(10, 1),表示该数组有10行,1列。
相关问题
请解释以下代码: data = np.loadtxt('data/{}.txt'.format(dataset)) n, _ = data.shape idx = np.array([i for i in range(n)], dtype=np.int32) idx_map = {j: i for i, j in enumerate(idx)} edges_unordered = np.genfromtxt(path, dtype=np.int32) edges = np.array(list(map(idx_map.get, edges_unordered.flatten())), dtype=np.int32).reshape(edges_unordered.shape) adj = sp.coo_matrix((np.ones(edges.shape[0]), (edges[:, 0], edges[:, 1])), shape=(n, n), dtype=np.float32)
这段代码用于读取一个文本文件,并生成一个稀疏矩阵,表示一个无向图的邻接矩阵。
具体解释如下:
1. `data = np.loadtxt('data/{}.txt'.format(dataset))`:读取文件,文件路径由变量`dataset`指定,将文件中的数据加载到一个 NumPy 数组 `data` 中。
2. `n, _ = data.shape`:获取 `data` 数组的形状,`n` 为数组的行数,表示节点的数量。
3. `idx = np.array([i for i in range(n)], dtype=np.int32)`:生成一个长度为 `n` 的一维数组 `idx`,其中每个元素代表一个节点的编号。
4. `idx_map = {j: i for i, j in enumerate(idx)}`:生成一个字典 `idx_map`,用于将节点编号映射为数组 `data` 中的行索引。
5. `edges_unordered = np.genfromtxt(path, dtype=np.int32)`:从文件中读取边的数据,将数据加载到一个 NumPy 数组 `edges_unordered` 中,每行代表一条边,每列为边的两个端点的节点编号。
6. `edges = np.array(list(map(idx_map.get, edges_unordered.flatten())), dtype=np.int32).reshape(edges_unordered.shape)`:将边的节点编号转换为数组 `data` 中的行索引,生成一个二维数组 `edges`,每行代表一条边,每列为边的两个端点的行索引。
7. `adj = sp.coo_matrix((np.ones(edges.shape[0]), (edges[:, 0], edges[:, 1])), shape=(n, n), dtype=np.float32)`:生成一个稀疏矩阵 `adj`,其中每个元素表示两个节点之间是否有边,如果有边则为 1,否则为 0。这里使用了 SciPy 中的 `coo_matrix` 函数,第一个参数是一个元组,表示每个非零元素的值和对应的行、列索引,第二个参数表示矩阵的形状,第三个参数表示数据类型。
import numpy as np import pickle import math f = open(r'C:\Users\sdnugeo\Desktop\target2.pkl','rb') bi = pickle.load(f) # bi = np.array(bi) data = np.arange(0, 156) print(data) data = data.tolist() # print(data) # 每次抽取1000个数值,共抽取10次 samples = [] c = [] a = 11 r = math.ceil(len(data)/a) print(r) for i in range(a): if len(data) > r : sample = np.random.choice(data, r, replace=False) else: sample = np.random.choice(data, len(data), replace=False) # for s in sample: # data.remove(s) # continue # print(type(sample)) # sample2 = np.array(sample) b = [bi[j] for j in list(sample)] # d = np.array(b) print(type(b)) c.append(b) # c = np.concatenate(c, axis=0) # c = bi[0] print(sample) # print(b) samples.append(sample) for s in sample: data.remove(s) # sample = [s for s in sample if s in data] samples = [np.array(s) for s in samples] samples = np.concatenate(samples, axis=0) c = [np.array(e) for e in c] c = np.concatenate(c, axis=0) # samples = np.array(samples).reshape(-1) print(samples) print(c.shape) 请帮我把这段代码修改成可调用的函数
好的,你想要将这段代码封装成一个函数,可以尝试以下的实现:
```python
import numpy as np
import pickle
import math
def sample_data(filepath):
f = open(filepath, 'rb')
bi = pickle.load(f)
data = np.arange(0, 156)
data = data.tolist()
samples = []
c = []
a = 11
r = math.ceil(len(data)/a)
for i in range(a):
if len(data) > r:
sample = np.random.choice(data, r, replace=False)
else:
sample = np.random.choice(data, len(data), replace=False)
b = [bi[j] for j in list(sample)]
c.append(b)
samples.append(sample)
for s in sample:
data.remove(s)
samples = [np.array(s) for s in samples]
samples = np.concatenate(samples, axis=0)
c = [np.array(e) for e in c]
c = np.concatenate(c, axis=0)
return samples, c
```
你可以将这个函数保存在一个.py文件中,然后在其他的python文件中,通过import导入并调用该函数。调用方法如下:
```python
from your_module import sample_data
filepath = r'C:\Users\sdnugeo\Desktop\target2.pkl'
samples, c = sample_data(filepath)
```
其中,filepath是你想要读取的pickle文件的路径。函数返回两个变量,分别为samples和c。
阅读全文
相关推荐
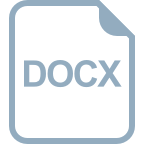
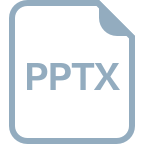
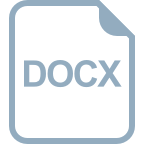













