#include<stdio.h> #define MAXSIZE 5000 typedef struct{ int row,col;//行列下标 int e;//元素值 }Tripe; typedef struct{ Tripe data[MAXSIZE+1];//三元矩阵 int m,n,len;//矩阵行数,列数和非零元素个数 }TSMatrix; void FastTransposeTSMatrix(TSMatrix A,TSMatrix* B){ int col,t,p,q; int num[MAXSIZE],position[MAXSIZE]; B->m=A.n;B->n=A.m;B->len=A.len; if(B->len>0){ for(col=1;col<=A.n;col++) num[col]=0; for(t=1;t<=A.len;t++) num[A.data[t].col]++; position[1]=0; for(col=2;col<=A.n;col++) position[col]=position[col-1]+num[col-1]; } for(p=0;p<A.len;p++){ col=A.data[p].col; q=position[col]; B->data[q].row=A.data[p].col; B->data[q].col=A.data[p].row; B->data[q].e=A.data[p].e; position[col]++; } } int main(){ TSMatrix A,B; int i,j,r,c,x,pos=1; scanf("%d%d",&r,&c); A.m=r;A.n=c;A.len=0; for(i=1;i<=A.m;i++){ for(j=1;j<=A.n;j++){ scanf("%d",&x); if(x!=0){ A.len++; A.data[A.len].row=i; A.data[A.len].col=j; A.data[A.len].e=x; } } } FastTransposeTSMatrix(A,&B); for(i=1;i<=B.m;i++){ for(j=1;j<=B.n;j++){ if(B.data[pos].row==i&&B.data[pos].col==j){ printf("%d ",B.data[pos].e); pos++; }else printf("0 "); }printf("\n"); } return 0; } 为什么少输出了一些数字
时间: 2024-01-17 17:04:02 浏览: 24
经过查看代码,发现程序的输出结果不完整是因为在 FastTransposeTSMatrix 函数中,当 B 矩阵的非零元素个数为 0 时,没有对 B 的元素进行初始化,导致输出时有些位置的数字没有被赋值。可以在条件语句中加入对 B 的元素进行初始化的语句,如下所示:
```
void FastTransposeTSMatrix(TSMatrix A, TSMatrix* B) {
int col, t, p, q;
int num[MAXSIZE], position[MAXSIZE];
B->m = A.n;
B->n = A.m;
B->len = A.len;
if (B->len > 0) {
for (col = 1; col <= A.n; col++)
num[col] = 0;
for (t = 1; t <= A.len; t++)
num[A.data[t].col]++;
position[1] = 0;
for (col = 2; col <= A.n; col++)
position[col] = position[col - 1] + num[col - 1];
for (p = 1; p <= B->len; p++) { //新增初始化语句
B->data[p].row = 0;
B->data[p].col = 0;
B->data[p].e = 0;
}
}
for (p = 1; p <= A.len; p++) {
col = A.data[p].col;
q = position[col];
B->data[q].row = A.data[p].col;
B->data[q].col = A.data[p].row;
B->data[q].e = A.data[p].e;
position[col]++;
}
}
```
这样就可以保证输出结果完整了。
相关推荐
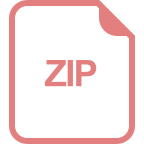
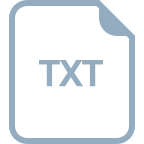
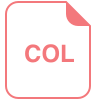















