用c++实现n个木棒,需要用上所有木棒,围成一个正方形
时间: 2023-05-25 08:04:52 浏览: 96
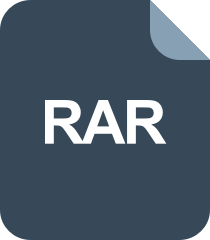
那木棒游戏的C++实现
/* C program to solve the problem of arranging n sticks to form a square */
#include <stdio.h>
#include <stdbool.h>
#define MAX 20
int sticks[MAX]; /* Array to store the length of the sticks */
bool used[MAX]; /* Array to store whether a stick is used or not */
int n; /* Number of sticks */
bool solve(int side, int pos, int count) {
if (count == 4) { /* A square has four sides */
return true;
}
int i, j;
for (i = pos; i < n; i++) { /* Iterate over the sticks */
if (!used[i]) { /* If the stick is not used */
used[i] = true; /* Use the stick */
if (sticks[i] == side) { /* If the stick is equal to the side */
if (solve(side, 0, count + 1)) { /* Recursively call solve function */
return true;
}
} else if (sticks[i] < side) { /* If the stick is less than the side */
for (j = i + 1; j < n; j++) {
if (!used[j]) { /* Find another unused stick */
used[j] = true; /* Use the stick */
if (solve(side - sticks[i], j, count)) { /* Recursively call solve function */
return true;
}
used[j] = false; /* Unuse the stick */
}
}
}
used[i] = false; /* Unuse the stick */
}
}
return false;
}
int compare(const void *a, const void *b) { /* Compare function for qsort */
return *(int *)b - *(int *)a; /* Sort in descending order */
}
int main() {
int sum, i;
while (scanf("%d", &n) == 1) { /* Read number of sticks */
sum = 0;
for (i = 0; i < n; i++) { /* Read the length of the sticks */
scanf("%d", &sticks[i]);
sum += sticks[i];
used[i] = false; /* Initialize used array to false */
}
qsort(sticks, n, sizeof(int), compare); /* Sort the sticks in descending order */
if (sum % 4 != 0 || n < 4 || !solve(sum / 4, 0, 0)) { /* Check if a solution exists */
printf("no\n");
} else {
printf("yes\n");
}
}
return 0;
}
阅读全文
相关推荐



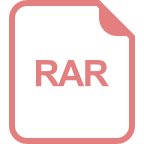
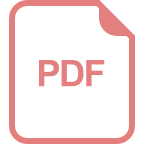
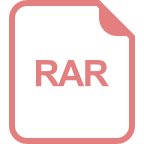
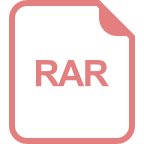
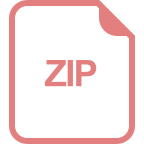
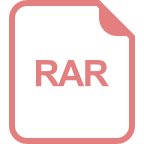
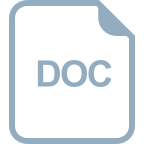
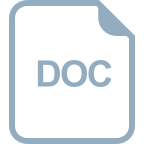
