稀疏矩阵的三元组顺序表示方法及基本操作的实现(建立、输出、转置)并实现一个主菜单来实现。实现稀疏矩阵的三元组表示下的普通转置、快速转置。
时间: 2024-05-03 09:16:29 浏览: 55
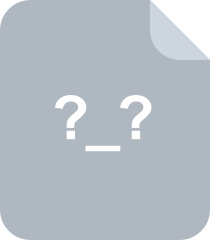
稀疏矩阵相加、相乘和转置(用三元组实现)

稀疏矩阵的三元组顺序表示方法:
在三元组顺序表中,每一个非零元素都由三个数据项表示:该元素的行下标、列下标和元素值。
在实现三元组顺序表示方法时,需要定义一个三元组结构体,如下所示:
```c++
struct Triple {
int row, col;
ElemType value;
};
```
其中,row表示元素所在行的行号,col表示元素所在列的列号,value表示元素的值。
基本操作的实现:
1. 建立稀疏矩阵的三元组表示方法:
```c++
void CreateSMatrix(TSMatrix &M) {
int row, col;
ElemType value;
cout << "请输入稀疏矩阵的行数、列数和非零元素个数:" << endl;
cin >> M.rows >> M.cols >> M.num;
cout << "请输入稀疏矩阵的非零元素(行、列、值):" << endl;
for (int i = 0; i < M.num; i++) {
cin >> row >> col >> value;
M.data[i].row = row;
M.data[i].col = col;
M.data[i].value = value;
}
}
```
2. 输出稀疏矩阵的三元组表示方法:
```c++
void PrintSMatrix(TSMatrix M) {
cout << "稀疏矩阵的三元组表示方法如下:" << endl;
cout << "行数:" << M.rows << " 列数:" << M.cols << " 非零元素个数:" << M.num << endl;
cout << "行 列 值" << endl;
for (int i = 0; i < M.num; i++) {
cout << M.data[i].row << " " << M.data[i].col << " " << M.data[i].value << endl;
}
}
```
3. 转置稀疏矩阵的三元组表示方法:
普通转置:
```c++
void TransposeSMatrix(TSMatrix M, TSMatrix &T) {
T.rows = M.cols;
T.cols = M.rows;
T.num = M.num;
if (T.num) {
int col, p;
vector<int> num(M.cols + 1, 0);
for (int i = 0; i < M.num; i++) {
col = M.data[i].col;
num[col]++;
}
vector<int> cpot(M.cols + 1, 0);
for (int i = 1; i <= M.cols; i++) {
cpot[i] = cpot[i - 1] + num[i - 1];
}
for (int i = 0; i < M.num; i++) {
col = M.data[i].col;
p = cpot[col];
T.data[p].row = M.data[i].col;
T.data[p].col = M.data[i].row;
T.data[p].value = M.data[i].value;
cpot[col]++;
}
}
}
```
快速转置:
```c++
void FastTransposeSMatrix(TSMatrix M, TSMatrix &T) {
T.rows = M.cols;
T.cols = M.rows;
T.num = M.num;
if (T.num) {
vector<int> num(M.cols + 1, 0);
for (int i = 0; i < M.num; i++) {
num[M.data[i].col]++;
}
vector<int> cpot(M.cols + 1, 0);
for (int i = 1; i <= M.cols; i++) {
cpot[i] = cpot[i - 1] + num[i - 1];
}
for (int i = 0; i < M.num; i++) {
int col = M.data[i].col;
int p = cpot[col];
T.data[p].row = M.data[i].col;
T.data[p].col = M.data[i].row;
T.data[p].value = M.data[i].value;
cpot[col]++;
}
}
}
```
主菜单的实现:
```c++
int main() {
TSMatrix M, T;
int choice;
while (true) {
cout << "请选择要进行的操作:" << endl;
cout << "1. 建立稀疏矩阵的三元组表示方法" << endl;
cout << "2. 输出稀疏矩阵的三元组表示方法" << endl;
cout << "3. 普通转置稀疏矩阵的三元组表示方法" << endl;
cout << "4. 快速转置稀疏矩阵的三元组表示方法" << endl;
cout << "5. 退出程序" << endl;
cin >> choice;
switch (choice) {
case 1:
CreateSMatrix(M);
break;
case 2:
PrintSMatrix(M);
break;
case 3:
TransposeSMatrix(M, T);
PrintSMatrix(T);
break;
case 4:
FastTransposeSMatrix(M, T);
PrintSMatrix(T);
break;
case 5:
exit(0);
default:
cout << "输入有误,请重新输入!" << endl;
}
}
return 0;
}
```
阅读全文
相关推荐



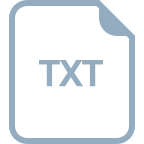
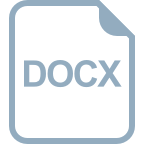
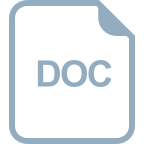
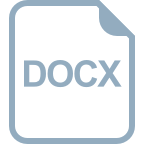
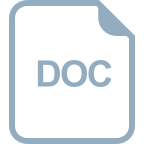
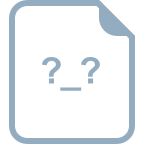
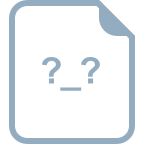