stm32f103zet6 使用串口2发送数据程序
时间: 2023-11-30 10:02:27 浏览: 39
以下是使用串口2发送数据的示例程序,假设波特率为115200:
```c
#include "stm32f10x.h"
void USART2_Init(void)
{
// 使能GPIOA时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 使能USART2时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
// 配置USART2的GPIO引脚
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2; // TX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; // 复用推挽输出
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; // GPIO速度50MHz
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART2
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200; // 波特率
USART_InitStructure.USART_WordLength = USART_WordLength_8b; // 数据位8位
USART_InitStructure.USART_StopBits = USART_StopBits_1; // 停止位1位
USART_InitStructure.USART_Parity = USART_Parity_No; // 无校验
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; // 无硬件流控制
USART_InitStructure.USART_Mode = USART_Mode_Tx; // 仅配置发送
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE); // 使能USART2
}
void USART2_SendChar(uint8_t ch)
{
// 等待发送缓冲区为空
while (USART_GetFlagStatus(USART2, USART_FLAG_TXE) == RESET);
USART_SendData(USART2, ch); // 发送数据
}
void USART2_SendString(const char *str)
{
while (*str)
{
USART2_SendChar(*str++);
}
}
int main(void)
{
USART2_Init();
while (1)
{
USART2_SendString("Hello, world!\r\n");
delay_ms(1000); // 延时1秒
}
}
```
程序中使用了 delay_ms 函数,需要自行实现。该函数的实现可以使用 SysTick 定时器,或者是其他的定时器实现。同时需要注意,该程序中只配置了 USART2 的发送功能,如果需要接收数据,还需要配置 USART2 的接收功能。
相关推荐
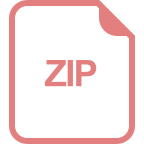
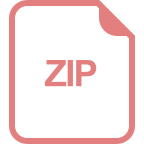














