用链表表示集合运算的代码 使用C++
时间: 2024-09-22 21:00:48 浏览: 37
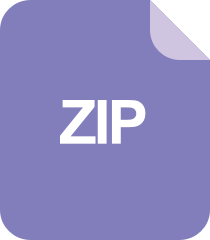
集合的运算(C++单链表实现).zip

链表可以用于模拟集合数据结构,特别是当我们需要频繁插入、删除元素,并保持元素的无序排列时,链表是一个不错的选择。这里有一个简单的例子,展示了如何使用C++的链表实现并集(Union)和交集(Intersection)操作:
```cpp
#include <iostream>
using namespace std;
// 链表节点
struct Node {
int value;
Node* next;
};
// 创建一个空链表
Node* createEmptyList() {
return new Node{0, nullptr};
}
// 插入值到链表
void insert(Node** head, int value) {
Node* newNode = new Node{value, nullptr};
if (*head == nullptr) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 并集操作
void unionSet(Node** set1, Node** set2) {
// 将set2的内容添加到set1
for (Node* node = *set2; node != nullptr; node = node->next) {
insert(set1, node->value);
}
}
// 交集操作
void intersectionSet(Node** set1, Node** set2) {
Node* current1 = *set1;
Node* current2 = *set2;
Node* result = createEmptyList(); // 新的结果链表
while (current1 != nullptr && current2 != nullptr) {
if (current1->value == current2->value) {
insert(&result, current1->value); // 如果值相同,添加到结果链表
}
if (current1->value < current2->value) {
current1 = current1->next;
} else {
current2 = current2->next;
}
}
*set1 = result; // 更新原set1为交集后的链表
}
// 打印链表
void printList(Node* head) {
while (head != nullptr) {
cout << head->value << " ";
head = head->next;
}
cout << endl;
}
int main() {
Node* set1 = createEmptyList();
Node* set2 = createEmptyList();
// 假设已经向set1和set2插入了一些元素...
insert(&set1, 1);
insert(&set1, 3);
insert(&set2, 2);
insert(&set2, 4);
unionSet(&set1, &set2); // set1现在包含[1, 2, 3, 4]
intersectionSet(&set1, &set2); // set1现在包含[2, 3]
cout << "并集后的set1: ";
printList(set1);
cout << "交集后的set1: ";
printList(set1);
return 0;
}
```
在这个例子中,`unionSet`函数合并了两个链表,而`intersectionSet`函数创建了一个新链表,其中包含了两个链表共享的所有元素。注意,这只是一个基本实现,实际应用中可能需要考虑更多的细节,如优化内存分配和处理空链表等。
阅读全文
相关推荐
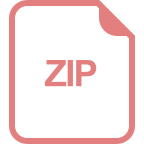
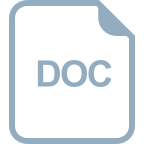
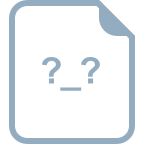
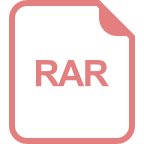
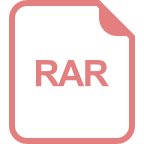
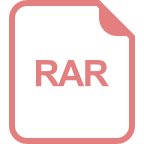
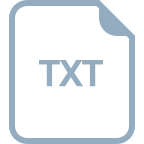
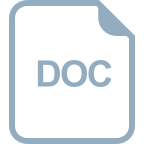
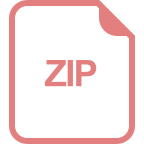
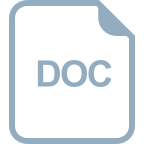
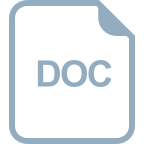
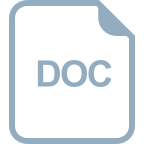
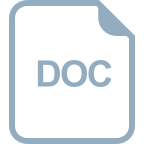
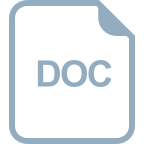
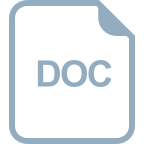


