使用numpy给出一个实现遗传算法的代码示例
时间: 2023-08-31 20:42:23 浏览: 63
### 回答1:
以下是一个简单的遗传算法示例代码,使用 numpy 库:
```python
import numpy as np
# 目标函数
def target_function(x):
return x**2
# 选择算子
def selection(pop, fitness):
idx = np.random.choice(np.arange(pop.shape[0]), size=pop.shape[0], replace=True, p=fitness/fitness.sum())
return pop[idx]
# 交叉算子
def crossover(pop, pc):
for i in range(0, pop.shape[0], 2):
if np.random.rand() < pc:
cpoint = np.random.randint(1, pop.shape[1])
temp1 = np.hstack((pop[i, :cpoint], pop[i+1, cpoint:]))
temp2 = np.hstack((pop[i+1, :cpoint], pop[i, cpoint:]))
pop[i], pop[i+1] = temp1, temp2
return pop
# 变异算子
def mutation(pop, pm):
for i in range(pop.shape[0]):
if np.random.rand() < pm:
mpoint = np.random.randint(0, pop.shape[1])
pop[i, mpoint] = np.random.randint(0, pop.shape[1])
return pop
# 遗传算法主体
def genetic_algorithm(pop_size, pc, pm, generations):
# 初始化种群
pop = np.random.randint(0, pop_size, size=(pop_size, 1))
# 进行迭代
for i in range(generations):
# 计算适应度
fitness = target_function(pop)
# 选择
pop = selection(pop, fitness)
# 交叉
pop = crossover(pop, pc)
# 变异
pop = mutation(pop, pm)
return pop
# 算法调用
best_solution = genetic_algorithm(pop_size=50, pc=0.8, pm=0.1, generations=100)
print(best_solution)
```
上面代码中,使用了一个简单的目标函数: x^2 。种群大小是50,交叉概率是0.8,变异概率是0.1,进化代数是100。
注意:这只是一个简单的示例
### 回答2:
以下是一个使用numpy实现遗传算法的简单代码示例:
import numpy as np
# 设置遗传算法的参数
population_size = 50 # 种群大小
chromosome_length = 10 # 染色体长度
mutation_rate = 0.02 # 变异率
crossover_rate = 0.6 # 交叉率
elitism_rate = 0.1 # 精英保留率
max_generations = 100 # 最大迭代次数
# 初始化种群
population = np.random.randint(low=0, high=2, size=(population_size, chromosome_length))
# 定义适应度函数
def fitness(chromosome):
return np.sum(chromosome)
# 主循环
for generation in range(max_generations):
# 计算适应度
fitness_scores = np.apply_along_axis(fitness, axis=1, arr=population)
# 精英保留
elite_size = int(population_size * elitism_rate)
elite_indices = np.argsort(fitness_scores)[-elite_size:]
elite_population = population[elite_indices]
# 选择、交叉、变异
selected_indices = np.random.choice(population_size, size=population_size - elite_size, replace=True, p=fitness_scores / np.sum(fitness_scores))
selected_population = population[selected_indices]
crossover_indices = np.random.choice(population_size - elite_size, size=int((population_size - elite_size) * crossover_rate / 2) * 2, replace=False)
crossover_population = selected_population[crossover_indices]
for i in range(0, crossover_population.shape[0], 2):
crossover_point = np.random.randint(low=1, high=chromosome_length)
crossover_population[i, crossover_point:] = selected_population[crossover_indices[i + 1], crossover_point:]
crossover_population[i + 1, crossover_point:] = selected_population[crossover_indices[i], crossover_point:]
mutation_indices = np.random.choice(population_size - elite_size, size=int((population_size - elite_size) * chromosome_length * mutation_rate), replace=False)
mutation_population = crossover_population[mutation_indices]
mutation_population[np.arange(len(mutation_indices)), np.random.randint(low=0, high=chromosome_length, size=mutation_indices.shape)] = 1 - mutation_population[np.arange(len(mutation_indices)), np.random.randint(low=0, high=chromosome_length, size=mutation_indices.shape)]
# 生成新的种群
population = np.concatenate((elite_population, mutation_population))
# 输出最终结果
best_fitness = np.max(np.apply_along_axis(fitness, axis=1, arr=population))
best_chromosome = population[np.argmax(np.apply_along_axis(fitness, axis=1, arr=population))]
print("最好的适应度:", best_fitness)
print("最好的染色体:", best_chromosome)
这个示例使用numpy来进行遗传算法的实现。首先是初始化种群,然后定义适应度函数。接着使用主循环来进行适应度计算、精英保留、选择、交叉和变异等操作。最后生成新的种群并输出最好的适应度和染色体。这只是一个简单的示例,实际应用中可能需要根据具体问题进行适当修改和优化。
### 回答3:
遗传算法是一种进化搜索算法,用于求解优化问题。下面是使用numpy库实现遗传算法的示例代码:
```python
import numpy as np
# 初始化种群
def initialize_population(population_size, chromosome_length):
return np.random.randint(2, size=(population_size, chromosome_length))
# 计算适应度
def calculate_fitness(population):
return np.sum(population, axis=1)
# 选择操作
def selection(population, fitness):
idx = np.random.choice(len(population), size=len(population), p=fitness/np.sum(fitness))
return population[idx]
# 交叉操作
def crossover(parents):
children = np.empty_like(parents)
crossover_points = np.random.randint(low=1, high=len(parents[0])-1, size=len(parents))
for i, point in enumerate(crossover_points):
children[i] = np.concatenate((parents[i][:point], parents[(i+1)%len(parents)][point:]))
return children
# 变异操作
def mutation(population, mutation_rate):
for i in range(len(population)):
for j in range(len(population[i])):
if np.random.random() < mutation_rate:
population[i][j] = 1 - population[i][j]
return population
# 主函数
def genetic_algorithm(population_size, chromosome_length, generations, mutation_rate):
population = initialize_population(population_size, chromosome_length)
for _ in range(generations):
fitness = calculate_fitness(population)
selected_parents = selection(population, fitness)
children = crossover(selected_parents)
mutated_children = mutation(children, mutation_rate)
population = mutated_children
return population
# 示例用法
population_size = 50
chromosome_length = 10
generations = 100
mutation_rate = 0.01
result_population = genetic_algorithm(population_size, chromosome_length, generations, mutation_rate)
print(result_population)
```
以上代码定义了一个简单的遗传算法,其中使用了numpy库的随机数生成、矩阵运算等功能来实现遗传算法的基本操作。算法根据给定的种群大小、染色体长度、迭代代数和变异率,返回最终的种群结果。在示例中,种群大小为50,染色体长度为10,迭代100代,变异率为0.01。运行示例代码后,将输出最终的种群结果。
相关推荐
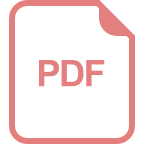
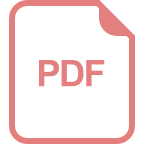














