在python中用遗传算法求解传教士和野人渡河问题
时间: 2023-09-22 12:15:25 浏览: 98
传教士和野人渡河问题是一个经典的人工智能问题,可以用遗传算法来求解。下面是一个简单的 Python 实现:
首先,我们需要定义问题的状态和操作。在这个问题中,状态包括当前河岸上的传教士和野人数量,以及船只的位置;操作包括将传教士或野人从一个河岸移动到另一个河岸。
```python
import random
class State:
def __init__(self, left_m, left_c, right_m, right_c, boat):
self.left_m = left_m # 左岸传教士数量
self.left_c = left_c # 左岸野人数量
self.right_m = right_m # 右岸传教士数量
self.right_c = right_c # 右岸野人数量
self.boat = boat # 船只位置:0 表示在左岸,1 表示在右岸
def is_valid(self):
if self.left_m < 0 or self.left_c < 0 or self.right_m < 0 or self.right_c < 0:
return False
if self.left_m > 0 and self.left_m < self.left_c:
return False
if self.right_m > 0 and self.right_m < self.right_c:
return False
return True
def move(self, m, c):
if self.boat == 0:
return State(self.left_m - m, self.left_c - c, self.right_m + m, self.right_c + c, 1)
else:
return State(self.left_m + m, self.left_c + c, self.right_m - m, self.right_c - c, 0)
def __str__(self):
return f"({self.left_m}, {self.left_c}, {self.right_m}, {self.right_c}, {self.boat})"
```
接下来,我们需要定义遗传算法的种群和适应度函数。在这个问题中,一个个体就是一系列操作序列,我们通过随机生成一些操作序列来初始化种群。适应度函数就是求解这个操作序列所对应的最短路径长度。
```python
POPULATION_SIZE = 100
NUM_GENERATIONS = 1000
def generate_population():
return [[random.randint(0, 2), random.randint(0, 2)] for _ in range(POPULATION_SIZE)]
def fitness(individual):
state = State(3, 3, 0, 0, 0)
path_length = 0
for action in individual:
new_state = state.move(*action)
if new_state.is_valid():
state = new_state
path_length += 1
else:
path_length += 10 # 惩罚非法状态
return 1 / (path_length + 1)
```
最后,我们可以运行遗传算法来求解最短路径:
```python
def evolve():
population = generate_population()
for generation in range(NUM_GENERATIONS):
fitnesses = [fitness(individual) for individual in population]
print(f"Generation {generation}: best fitness = {max(fitnesses)}")
# 选择
selected_indices = random.choices(range(POPULATION_SIZE), weights=fitnesses, k=POPULATION_SIZE)
# 交叉
offspring = []
for i in range(0, POPULATION_SIZE, 2):
parent1 = population[selected_indices[i]]
parent2 = population[selected_indices[i + 1]]
child1 = [parent1[0], parent2[1]]
child2 = [parent2[0], parent1[1]]
offspring.extend([child1, child2])
# 变异
for i in range(POPULATION_SIZE):
if random.random() < 0.1:
offspring[i][0] = random.randint(0, 2)
if random.random() < 0.1:
offspring[i][1] = random.randint(0, 2)
population = offspring
best_individual = max(population, key=fitness)
print(f"Best individual: {best_individual}, fitness = {fitness(best_individual)}")
```
这个遗传算法的实现非常简单,可以通过增加种群大小和迭代次数来提高求解的精度。
阅读全文
相关推荐
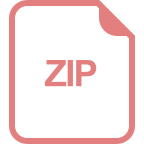
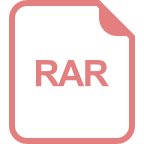















