x = x[(x[:, 5:6] == torch.tensor(classes, device=x.device)).any(1)]
时间: 2024-05-26 12:15:39 浏览: 104
这行代码的作用是从张量x中筛选出第6列元素中包含给定类别(classes)的行,并将结果重新赋值给x。具体来说,它首先通过比较x的第6列元素与给定类别(classes)是否相等,得到一个布尔值的张量,再使用any(1)函数沿着第1维(即行)的方向取或运算,得到一个新的布尔值的张量,表示哪些行中至少有一个元素等于给定类别。最后,使用这个布尔值张量对x进行索引,得到符合条件的行。
相关问题
class MLP(nn.Module): def __init__( self, input_size: int, output_size: int, n_hidden: int, classes: int, dropout: float, normalize_before: bool = True ): super(MLP, self).__init__() self.input_size = input_size self.dropout = dropout self.n_hidden = n_hidden self.classes = classes self.output_size = output_size self.normalize_before = normalize_before self.model = nn.Sequential( nn.Linear(self.input_size, n_hidden), nn.Dropout(self.dropout), nn.ReLU(), nn.Linear(n_hidden, self.output_size), nn.Dropout(self.dropout), nn.ReLU(), ) self.after_norm = torch.nn.LayerNorm(self.input_size, eps=1e-5) self.fc = nn.Sequential( nn.Dropout(self.dropout), nn.Linear(self.input_size, self.classes) ) self.output_layer = nn.Linear(self.output_size, self.classes) def forward(self, x): self.device = torch.device('cuda') # x = self.model(x) if self.normalize_before: x = self.after_norm(x) batch_size, length, dimensions = x.size(0), x.size(1), x.size(2) output = self.model(x) return output.mean(dim=1) class LabelSmoothingLoss(nn.Module): def __init__(self, size: int, smoothing: float, ): super(LabelSmoothingLoss, self).__init__() self.size = size self.criterion = nn.KLDivLoss(reduction="none") self.confidence = 1.0 - smoothing self.smoothing = smoothing def forward(self, x: torch.Tensor, target: torch.Tensor) -> torch.Tensor: batch_size = x.size(0) if self.smoothing == None: return nn.CrossEntropyLoss()(x, target.view(-1)) true_dist = torch.zeros_like(x) true_dist.fill_(self.smoothing / (self.size - 1)) true_dist.scatter_(1, target.view(-1).unsqueeze(1), self.confidence) kl = self.criterion(torch.log_softmax(x, dim=1), true_dist) return kl.sum() / batch_size
这段代码中定义了一个 MLP 模型以及一个 LabelSmoothingLoss 损失函数。MLP 模型包含了多个线性层和 ReLU 激活函数,以及一个 LayerNorm 层和一个 dropout 层。LabelSmoothingLoss 损失函数主要用于解决分类问题中的过拟合问题,它通过对真实标签进行平滑处理来减少模型对噪声的敏感度。这段代码的 forward 方法实现了 MLP 模型的前向传播,以及 LabelSmoothingLoss 的计算。其中,true_dist 是经过平滑处理后的真实标签分布,kl 是计算 KL 散度的结果,最终返回的是 kl 的平均值。
如何将其中测试集的loss函数收敛:class MLP(torch.nn.Module): def init(self, weight_decay=0.01): super(MLP, self).init() self.fc1 = torch.nn.Linear(178, 100) self.relu = torch.nn.ReLU() self.fc2 = torch.nn.Linear(100, 50) self.fc3 = torch.nn.Linear(50, 5) self.dropout = torch.nn.Dropout(p=0.1) self.weight_decay = weight_decay def forward(self, x): x = self.fc1(x) x = self.relu(x) x = self.fc2(x) x = self.relu(x) x = self.fc3(x) return x def regularization_loss(self): reg_loss = torch.tensor(0.).to(device) for name, param in self.named_parameters(): if 'weight' in name: reg_loss += self.weight_decay * torch.norm(param) return reg_lossmodel = MLP() criterion = torch.nn.CrossEntropyLoss() optimizer = torch.optim.Adam(model.parameters(), lr=0.001) for epoch in range(num_epochs): for i, (inputs, labels) in enumerate(train_loader): optimizer.zero_grad() outputs = model(inputs.to(device)) loss = criterion(outputs, labels.to(device)) loss += model.regularization_loss() loss.backward() optimizer.step()
根据给出的代码,下列是一些建议来使该模型的测试集的损失函数收敛:
1. 增加训练周期(num_epochs),以便为模型提供更多的训练时间;
2. 减小 Adam 优化器的学习率(lr),以防止步长过大;
3. 增加批量大小(batch_size),以减少计算中的噪声;
4. 在模型中增加 Batch Normalization 层,以确保模型更稳定地收敛;
5. 增加模型的复杂度,例如增加层数或增加节点数。
这是一些可能有用的泛化策略,并不能直接保证损失函数能够收敛。在实践中,我们应该根据对数据的理解和实验结果来调整这些超参数,以便使模型更准确地进行预测。
阅读全文
相关推荐
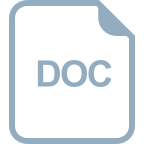
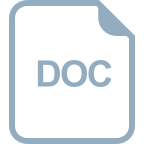
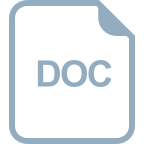













