C语言实现约瑟夫环问题:N个人围成一圈,从第一个开始报数,第M个将被杀掉,最后剩下一个,其余人都将被杀掉。例如N=6,M=5,被杀的顺序是:5,4,6,2,3,1。输入两个正整数N和M,N表示N个人,M表示报数到M;输出依次出列的序号。以空格作为分隔。用循环链表实现,补充函数内容实现程序要求。
时间: 2023-05-30 15:05:05 浏览: 144
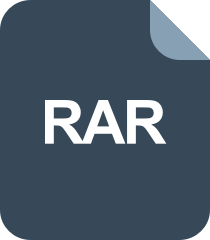
C语言“约瑟夫环”问题实现
```c
#include <stdio.h>
#include <stdlib.h>
struct Node{
int data;
struct Node *next;
};
typedef struct Node *PtrToNode;
typedef PtrToNode List;
typedef PtrToNode Position;
List CreateList(int N); //创建循环链表
void Josephus(List L, int M); //约瑟夫环问题
int main()
{
int N, M;
scanf("%d %d", &N, &M);
List L = CreateList(N);
Josephus(L, M);
return 0;
}
List CreateList(int N)
{
List L = (List)malloc(sizeof(struct Node));
L->next = L;
Position P = L;
for(int i = 1; i <= N; i++){
Position tmp = (Position)malloc(sizeof(struct Node));
tmp->data = i;
tmp->next = P->next;
P->next = tmp;
P = P->next;
}
return L;
}
void Josephus(List L, int M)
{
Position P = L;
while(P->next != P){
for(int i = 1; i < M; i++){
P = P->next;
}
Position tmp = P->next;
printf("%d ", tmp->data);
P->next = tmp->next;
free(tmp);
}
printf("%d\n", P->data);
free(P);
}
```
阅读全文
相关推荐
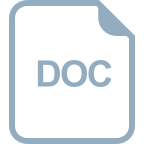



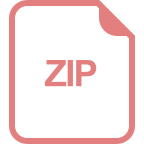


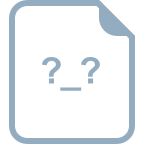
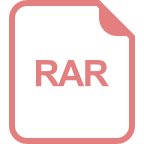
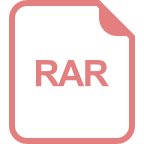
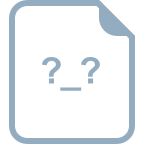