python django 多线程
时间: 2024-07-22 16:01:27 浏览: 91
在Python Django中,多线程是一种并发处理机制,它允许应用程序同时执行多个任务,而不会阻塞主线程。Django本身并不直接提供多线程支持,因为其底层使用的是异步I/O模型(如Asynchronous IO或Gevent),这更适合Web服务器环境,因为它避免了线程安全问题。
然而,如果你想在Django应用中手动实现多线程,可以借助Python标准库的`threading`模块。例如,你可以创建一个新的线程来执行某个耗时的操作,而不是阻塞整个请求处理。下面是一个简单的例子:
```python
from threading import Thread
def my_threaded_function():
# 这里放置你的耗时操作
pass
def view_func(request):
thread = Thread(target=my_threaded_function)
thread.start() # 开始新线程
# 继续执行视图函数的其他部分,主线程不会等待线程完成
# 返回响应等...
相关问题
django多线程处理请求
在 Django 中处理多线程请求,可以使用 Python 内置的 threading 模块或者使用 Django 自带的线程池 ThreadPoolExecutor。
使用 threading 模块:
1. 在视图函数中创建线程,例如:
```
import threading
def my_view(request):
t = threading.Thread(target=long_running_job)
t.start()
return HttpResponse('Thread started')
```
2. 在线程中处理长时间运行的任务:
```
def long_running_job():
# do something that takes a long time
```
使用 ThreadPoolExecutor:
1. 安装 concurrent.futures 包。
2. 在 settings.py 中配置线程池大小:
```
CONCURRENT_REQUESTS = 8 # 设置线程池大小
```
3. 在视图函数中使用线程池:
```
from concurrent.futures import ThreadPoolExecutor
executor = ThreadPoolExecutor(CONCURRENT_REQUESTS)
def my_view(request):
executor.submit(long_running_job)
return HttpResponse('Thread started')
```
4. 在线程中处理长时间运行的任务:
```
def long_running_job():
# do something that takes a long time
```
需要注意的是,使用多线程处理请求可能会带来一些风险和不确定性,需要谨慎使用,特别是在涉及到访问共享资源或者并发性能问题的情况下。
python web 多线程
Python中可以使用多种方式实现Web多线程,以下是一些常用的方法:
1. 使用 threading 模块:Python标准库中的 threading 模块提供了多线程的支持。你可以创建多个线程来处理Web请求并发的情况。例如:
```python
import threading
from http.server import HTTPServer, BaseHTTPRequestHandler
class MyHandler(BaseHTTPRequestHandler):
def do_GET(self):
# 处理GET请求的逻辑
...
def run_server():
server_address = ('', 8000)
httpd = HTTPServer(server_address, MyHandler)
httpd.serve_forever()
# 创建多个线程来处理请求
for _ in range(5):
t = threading.Thread(target=run_server)
t.start()
```
2. 使用 asyncio 模块:Python 3.4及以上版本引入了 asyncio 模块,提供了基于协程的异步编程。你可以使用 asyncio 来处理Web请求并发的情况。例如:
```python
import asyncio
from aiohttp import web
async def handle(request):
# 处理请求的逻辑
...
app = web.Application()
app.router.add_get('/', handle)
# 运行多个协程来处理请求
for _ in range(5):
asyncio.ensure_future(web.run_app(app))
```
3. 使用第三方框架:还有一些第三方框架(如 Flask、Django)提供了对多线程的支持,你可以根据具体需求选择适合的框架。
请注意,多线程并不一定能带来性能的提升,具体的并发处理方式需要根据实际情况来选择。另外,在多线程编程中要注意线程安全和资源争用的问题。
相关推荐
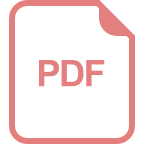
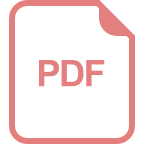
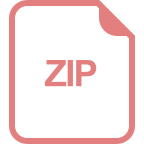
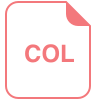
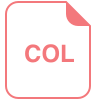
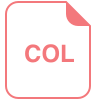
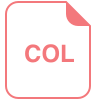
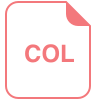







