用java写一个银行家算法
时间: 2023-05-19 07:04:13 浏览: 114
银行家算法是一种避免死锁的算法,它可以确保系统中的资源分配是安全的。以下是一个用 Java 实现银行家算法的示例代码:
```
import java.util.*;
public class BankerAlgorithm {
private int[][] need;
private int[][] allocation;
private int[] available;
private int[] safeSequence;
private int numOfProcesses;
private int numOfResources;
public BankerAlgorithm(int[][] need, int[][] allocation, int[] available) {
this.need = need;
this.allocation = allocation;
this.available = available;
this.numOfProcesses = need.length;
this.numOfResources = available.length;
this.safeSequence = new int[numOfProcesses];
}
public boolean isSafe() {
boolean[] finish = new boolean[numOfProcesses];
int[] work = Arrays.copyOf(available, numOfResources);
int count = 0;
while (count < numOfProcesses) {
boolean found = false;
for (int i = 0; i < numOfProcesses; i++) {
if (!finish[i] && Arrays.equals(need[i], Arrays.copyOfRange(work, 0, numOfResources))) {
finish[i] = true;
work = addArrays(work, allocation[i]);
safeSequence[count] = i;
count++;
found = true;
}
}
if (!found) {
return false;
}
}
return true;
}
public int[] getSafeSequence() {
if (isSafe()) {
return safeSequence;
} else {
return null;
}
}
private int[] addArrays(int[] a, int[] b) {
int[] result = new int[a.length];
for (int i = 0; i < a.length; i++) {
result[i] = a[i] + b[i];
}
return result;
}
}
```
这个类接受三个参数:need、allocation 和 available。need 和 allocation 都是二维数组,表示每个进程需要和已经分配的资源数量。available 是一个一维数组,表示当前可用的资源数量。isSafe() 方法用于检查当前状态是否安全,getSafeSequence() 方法返回一个安全序列(如果存在)。
注意:这只是一个简单的实现,实际上银行家算法还有很多细节需要考虑,比如资源请求的顺序、死锁检测等。
阅读全文
相关推荐
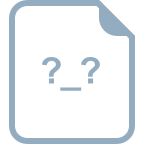
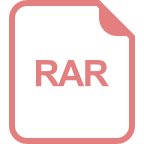
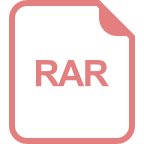
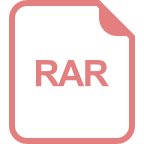








