写一段随机森林的python代码
时间: 2023-05-25 17:03:48 浏览: 62
这是一段简单的随机森林的Python代码:
```
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import make_classification
# 生成随机数据集作为例子
X, y = make_classification(n_samples=1000, n_features=4,
n_informative=2, n_redundant=0,
random_state=0, shuffle=False)
# 定义随机森林模型
clf = RandomForestClassifier(n_estimators=100, max_depth=2,
random_state=0)
# 训练模型
clf.fit(X, y)
# 使用模型进行预测
print(clf.predict([[0, 0, 0, 0]]))
```
上面的代码中,我们首先使用`make_classification`函数生成了一个含有4个特征和1000个样本的数据集,然后定义了一个随机森林分类器,包含100棵树,每棵树的深度不超过2。最后,我们使用`fit`方法训练了模型,并用`predict`方法对一个新样本做出了预测。
相关问题
写一段随机森林回归python代码
以下是一个简单的随机森林回归Python代码:
```python
from sklearn.ensemble import RandomForestRegressor
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# 加载波士顿房价数据集
boston = load_boston()
# 分割训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(boston.data, boston.target, test_size=0.2, random_state=42)
# 创建随机森林回归模型
rf_regressor = RandomForestRegressor(n_estimators=100, random_state=42)
# 训练模型
rf_regressor.fit(X_train, y_train)
# 预测测试集结果
y_predicted = rf_regressor.predict(X_test)
# 计算均方误差
mse = mean_squared_error(y_test, y_predicted)
print("均方误差:", mse)
```
在这个示例中,我们使用了Scikit-learn中的随机森林回归模型,并使用波士顿房价数据集进行训练和测试。我们将训练集分割为80%的训练数据和20%的测试数据。我们使用100个决策树来构建随机森林模型,并计算测试数据的均方误差。
用python写一段随机森林分类代码
以下是用Python编写的随机森林分类代码:
```
# 导入必要的库
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
# 生成随机数据集
X, y = make_classification(n_samples=1000, n_features=4, random_state=0, shuffle=False)
# 将数据集拆分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.33, random_state=42)
# 创建随机森林分类器对象
clf = RandomForestClassifier(n_estimators=100, max_depth=2, random_state=0)
# 训练模型
clf.fit(X_train, y_train)
# 预测测试集
y_pred = clf.predict(X_test)
# 输出模型得分
print(clf.score(X_test, y_test))
```
希望这段代码对你有所帮助!
相关推荐
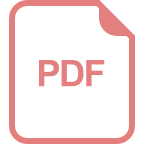
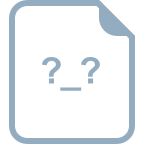












