C++用类和对象编写生命游戏
时间: 2024-01-18 09:03:31 浏览: 25
下面是使用类和对象编写生命游戏的C++代码:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <unistd.h>
using namespace std;
const int ROW = 20;
const int COL = 50;
class Cell {
public:
Cell();
void set_state(int state);
int get_state() const;
void set_next_state(int state);
int get_next_state() const;
int count_neighbors(const Cell** world, int row, int col) const;
private:
int state_;
int next_state_;
};
Cell::Cell() {
state_ = rand() % 2;
}
void Cell::set_state(int state) {
state_ = state;
}
int Cell::get_state() const {
return state_;
}
void Cell::set_next_state(int state) {
next_state_ = state;
}
int Cell::get_next_state() const {
return next_state_;
}
int Cell::count_neighbors(const Cell** world, int row, int col) const {
int count = 0;
for (int i = row - 1; i <= row + 1 ; i++) {
for (int j = col - 1; j <= col + 1; j++) {
if (i == row && j == col) {
continue;
}
int r = (i + ROW) % ROW;
int c = (j + COL) % COL;
count += world[r][c].get_state();
}
}
return count;
}
class World {
public:
World();
void init();
void print() const;
void next();
private:
Cell cells_[ROW][COL];
};
World::World() {}
void World::init() {
srand(time(NULL));
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
cells_[i][j].set_state(rand() % 2);
}
}
}
void World::print() const {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (cells_[i][j].get_state() == 1) {
cout << "*";
} else {
cout << " ";
}
}
cout << endl;
}
}
void World::next() {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
int neighbors = cells_[i][j].count_neighbors((const Cell**)cells_, i, j);
if (cells_[i][j].get_state() == 1) {
if (neighbors == 2 || neighbors == 3) {
cells_[i][j].set_next_state(1);
} else {
cells_[i][j].set_next_state(0);
}
} else {
if (neighbors == 3) {
cells_[i][j].set_next_state(1);
} else {
cells_[i][j].set_next_state(0);
}
}
}
}
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
cells_[i][j].set_state(cells_[i][j].get_next_state());
}
}
}
int main() {
World world;
world.init();
while (true) {
world.print();
world.next();
usleep(100000);
system("clear");
}
return 0;
}
```
在这个实现中,我们定义了两个类:`Cell` 和 `World`。`Cell` 表示一个单独的方格,它有两个状态:当前状态和下一个状态。`World` 表示整个方格世界,它由多个 `Cell` 组成。
在 `Cell` 类中,我们实现了 `count_neighbors()` 函数来计算每个方格周围活着的方格数。在 `World` 类中,我们实现了 `init()` 函数来随机初始化方格世界,实现了 `print()` 函数来打印方格世界,实现了 `next()` 函数来根据生命游戏的规则计算下一次迭代的状态。
在主函数中,我们创建了一个 `World` 对象,并不断地打印方格世界,计算下一次迭代的状态,然后清空屏幕并休眠一段时间,以形成动画效果。
相关推荐
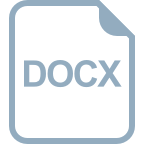
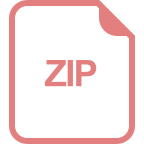
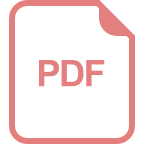














