#include <stdio.h> #include <stdlib.h> struct telnote { int no; char name[20]; char tel[20]; }; int main(void) { int code, i, cnt = 0; char scode[10]; FILE *fp; struct telnote mytel[10], buff, * p; p = &buff; while (1) { printf("\nコード入力:"); gets_s(scode); code = atoi(scode); if (code <= 0)break; p->no = code; printf("氏名入力:"); gets_s(p->name); printf("電話番号入力:"); gets_s(p->tel); mytel[cnt] = ;//补充完成 ++cnt; } if (cnt > 0) { fp = fopen("mytel.data", "w"); if (fp == NULL) { printf("file open error!!\n"); exit(1); } printf("\nコード氏名 電話番号\n"); for (i = 0; i < cnt; i++) { printf("%5d %-15s %-15s\n", buff.no, buff.name, buff.tel); fprintf();//补充完成 } fclose(fp); } else { printf("\nデータが入力されていません\n"); } return 0; }把缺失的补充完成
时间: 2024-03-29 15:36:00 浏览: 13
在代码中,需要补充完成以下两处:
1. 在while循环中,将输入的数据存入数组中。
```c
while (1) {
printf("\nコード入力:");
gets_s(scode);
code = atoi(scode);
if (code <= 0)break;
p->no = code;
printf("氏名入力:");
gets_s(p->name);
printf("電話番号入力:");
gets_s(p->tel);
mytel[cnt] = buff; // 将输入的数据存入数组中
++cnt;
}
```
2. 在for循环中,将数据写入文件中。
```c
for (i = 0; i < cnt; i++) {
printf("%5d %-15s %-15s\n", mytel[i].no, mytel[i].name, mytel[i].tel);
fprintf(fp, "%d %s %s\n", mytel[i].no, mytel[i].name, mytel[i].tel); // 将数据写入文件中
}
```
相关问题
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
#include <stdio.h> #include <stdlib.h>
#include <stdio.h>和#include <stdlib.h>是C语言中的两个头文件。
1. #include <stdio.h>是用来包含标准输入输出函数的头文件。它提供了一些用于输入和输出的函数,比如printf和scanf等。通过包含这个头文件,我们可以在程序中使用这些函数来进行输入和输出操作。
2. #include <stdlib.h>是用来包含标准库函数的头文件。它提供了一些常用的函数,比如内存分配函数malloc和free,字符串转换函数atoi和atof等。通过包含这个头文件,我们可以在程序中使用这些函数来进行各种操作。
相关推荐
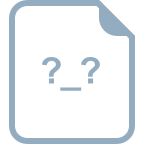
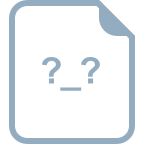
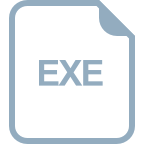













