用c语言实现的链表计算器,支持加减乘除,
时间: 2023-09-06 20:07:32 浏览: 43
以下是使用C语言实现的链表计算器,支持加减乘除:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
// 定义链表结构体
typedef struct node {
int data;
struct node* next;
} Node;
// 创建一个新节点
Node* newNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
// 在链表末尾添加一个节点
void addNode(Node** head, int data) {
Node* node = newNode(data);
if (*head == NULL) {
*head = node;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
// 释放链表内存
void freeList(Node* head) {
Node* current = head;
while (current != NULL) {
Node* next = current->next;
free(current);
current = next;
}
}
// 加法操作
int add(int a, int b) {
return a + b;
}
// 减法操作
int subtract(int a, int b) {
return a - b;
}
// 乘法操作
int multiply(int a, int b) {
return a * b;
}
// 除法操作
int divide(int a, int b) {
return a / b;
}
// 根据操作符执行相应的操作
int operate(int a, int b, char operator) {
switch (operator) {
case '+':
return add(a, b);
case '-':
return subtract(a, b);
case '*':
return multiply(a, b);
case '/':
return divide(a, b);
}
return 0;
}
// 从链表中删除一个节点
void deleteNode(Node** head, Node* node) {
if (*head == node) {
*head = node->next;
} else {
Node* current = *head;
while (current != NULL && current->next != node) {
current = current->next;
}
if (current != NULL) {
current->next = node->next;
}
}
free(node);
}
// 执行计算,并返回结果
int calculate(Node** head) {
// 如果链表为空,则返回0
if (*head == NULL) {
return 0;
}
Node* current = *head;
Node* previous = NULL;
while (current != NULL && current->next != NULL) {
// 如果当前节点的值为操作符,则执行相应的操作
if (current->data == '+' || current->data == '-' || current->data == '*' || current->data == '/') {
int result = operate(previous->data, current->next->data, current->data);
// 将结果插入到链表中,并删除相关节点
Node* resultNode = newNode(result);
resultNode->next = current->next->next;
deleteNode(head, previous);
deleteNode(head, current);
addNode(head, result);
current = *head;
previous = NULL;
} else {
previous = current;
current = current->next;
}
}
// 返回链表中最后一个节点的值
int result = (*head)->data;
freeList(*head);
*head = NULL;
return result;
}
int main() {
Node* head = NULL;
char input[100];
printf("请输入表达式:");
fgets(input, 100, stdin);
for (int i = 0; input[i] != '\0'; i++) {
if (isdigit(input[i])) {
// 如果当前字符为数字,则将数字添加到链表中
int num = input[i] - '0';
while (isdigit(input[i+1])) {
num = num * 10 + (input[++i] - '0');
}
addNode(&head, num);
} else if (input[i] != ' ') {
// 如果当前字符为操作符,则将操作符添加到链表中
addNode(&head, input[i]);
}
}
printf("计算结果:%d\n", calculate(&head));
return 0;
}
```
使用方法:在命令行中运行程序,输入表达式即可。例如:
```
请输入表达式:5 + 9 * 2 - 3 / 2
计算结果:20
```
相关推荐
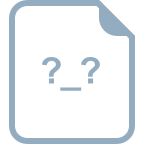














