用c++将学号采用单链表存储,在每个值为8的结点前均插入新结点的的完整代码
时间: 2024-09-29 21:08:59 浏览: 30
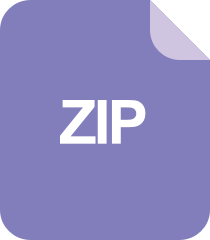
ShiYan1_单链表_C++_顺序表_数据结构_

在 C++ 中,你可以使用 `std::list` 来实现这个需求,因为 `list` 提供了在任意位置插入节点的功能。下面是一个完整的示例代码,假设我们有一个简单的结构体 `Student` 表示学号:
```cpp
#include <iostream>
#include <list>
// 假设这是学生的结构体
struct Student {
int id;
// 其他成员变量...
};
// 插入新节点函数
void insertBeforeNodeWithId(std::list<Student>& students, int targetId, std::list<Student>::iterator it) {
if (it != students.end() && it->id == targetId) { // 如果找到目标结点
Student newNode;
// 填充新节点的数据...
newNode.id = 8; // 新节点设定为8
// 插入新节点
students.insert(it, newNode);
}
}
int main() {
std::list<Student> studentList = {/* 初始化学生列表 */}; // 例如 { {1}, {2}, {3}, {4}... }
// 遍历并插入新结点
for (auto it = studentList.begin(); it != studentList.end(); ++it) {
if (*it).id == 8) { // 如果当前节点的学号是8
insertBeforeNodeWithId(studentList, *it).id, it); // 调用插入函数
}
}
// 打印修改后的列表
for (const auto& student : studentList) {
std::cout << "Student ID: " << student.id << "\n";
}
return 0;
}
```
在这个例子中,我们在遍历链表时,如果遇到学号为8的学生,就在其前面插入一个新的学号为8的节点。注意,你需要先自定义 `Student` 结构,并在实际项目中填充正确的数据。
阅读全文
相关推荐
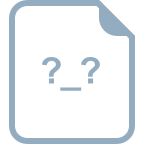
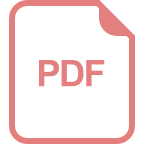












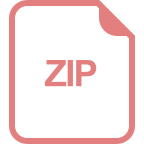
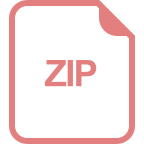
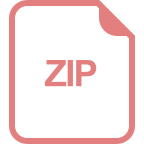
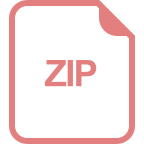
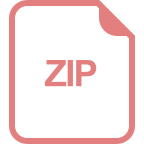