在上题基础上,输入一个学号,在单链表上查找该学号的结点,找到,则删除该结点,找不到,则给出提示。
时间: 2024-03-25 07:38:22 浏览: 22
好的,下面是代码实现:
```c++
#include <iostream>
#include <string.h>
using namespace std;
// 定义学生结构体
struct Student {
char id[10]; // 学号
char Name[20]; // 姓名
double Score[3]; // 3门课程成绩
Student* next; // 下一个结点指针
};
// 创建链表函数
Student* createList() {
// 创建头结点
Student* head = new Student;
head->next = NULL;
// 输入学生信息,创建新结点
int n;
cout << "请输入学生人数:";
cin >> n;
for (int i = 0; i < n; i++) {
// 创建新结点
Student* p = new Student;
// 输入学生信息
cout << "请输入第" << i+1 << "个学生的学号、姓名和3门课程成绩:";
cin >> p->id >> p->Name;
for (int j = 0; j < 3; j++) {
cin >> p->Score[j];
}
p->next = NULL;
// 将新结点插入链表尾部
Student* q = head;
while (q->next != NULL) {
q = q->next;
}
q->next = p;
}
return head;
}
// 查找并删除结点函数
bool deleteNode(Student* head, char* id) {
// 查找结点
Student* p = head->next;
Student* q = head;
while (p != NULL) {
if (strcmp(p->id, id) == 0) { // 找到
q->next = p->next; // 删除结点
delete p;
return true;
}
q = p;
p = p->next;
}
// 没找到
return false;
}
// 输出链表函数
void printList(Student* head) {
Student* p = head->next;
while (p != NULL) {
cout << p->id << " " << p->Name << " ";
for (int i = 0; i < 3; i++) {
cout << p->Score[i] << " ";
}
cout << endl;
p = p->next;
}
}
int main() {
// 创建链表
Student* head = createList();
// 输出链表
cout << "原链表:" << endl;
printList(head);
// 查找并删除结点
char id[10];
cout << "请输入要删除的学生的学号:";
cin >> id;
bool isFound = deleteNode(head, id);
if (isFound) {
cout << "删除成功!" << endl;
} else {
cout << "未找到该学生!" << endl;
}
// 输出链表
cout << "新链表:" << endl;
printList(head);
return 0;
}
```
运行程序后,输入学生信息,然后输入要删除的学生的学号,程序会在链表上查找该学号的结点,如果找到,则删除该结点,并输出“删除成功!”;否则输出“未找到该学生!”。最后输出删除结点后的链表。
相关推荐
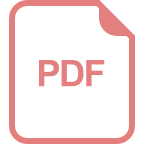














